Backend APIs
Connect your database / APIs and write business logic in JavaScript or Python. Reference previous steps and components to make your application dynamic.
What are Superblocks APIs?
Superblocks APIs are the business logic layer of your application, built on top of your data sources. These backend APIs run server side (on Superblocks servers if you're using the cloud version or on your own infrastructure if you're using the On-premise Agent), and send a response back to your components in the frontend user interface. This separation of the application into a frontend and backend is a core concept when working with APIs. For example, a backend API cannot update a frontend component's state directly. Instead, the component reacts to the response from the API.
In the example below, we query the Zendesk GET Ticket API, then get the associated customer orders from Postgres, get the order shipping data from Snowflake, and merge and transform the Postgres/Snowflake output using Python.
Working with Superblocks APIs
Referencing variables in backend APIs
Inside backend API steps, you can use existing variables to reference:
- Frontend component properties and frontend variables
- Output of previous steps in the current API
- Responses from other APIs
When referencing any of these variables in a backend API, put the content inside of {{}}
bindings, except for JavaScript and Python steps. For language steps, bindings are not needed.
Referencing frontend component properties and frontend variables
You can reference frontend component properties and frontend variables in backend API steps. For example, filter a SQL statement by passing in the value typed into an Input component:
Examples of other component properties:
Input1.text //The value of the input
Table1.selectedRow.city //The selected row JSON object from the Table
Chart1.selectedData //The selected data point JSON object from the Chart
Dropdown1.selectedOptionValue //The option selected in the Dropdown
The same can be applied for frontend variables, by referencing STATE_VAR_NAME.value
in a backend API.
Referencing a previous step output in the API builder
You can also reference the output from previous steps in an API.
Examples of step output properties:
Step1.output //The JSON output object from the Step1
Step1.output[0] //The object at index 0 of the array
Step1.output[0].id //The id property of the 0th index
Referencing another API response in the API builder
The output of a standard synchronous API can be referenced within an API step. Note, the referenced API must run before its value is referenced. In the example below, the response from API1
is referenced in API2
:
Running APIs and single steps
In the backend API editor, you can run the entire API with the Run API button, or run individual steps with the Test Step button. The latter makes it easier to iterate on APIs and test step changes as you go, without needing to unnecessarily run other steps again.
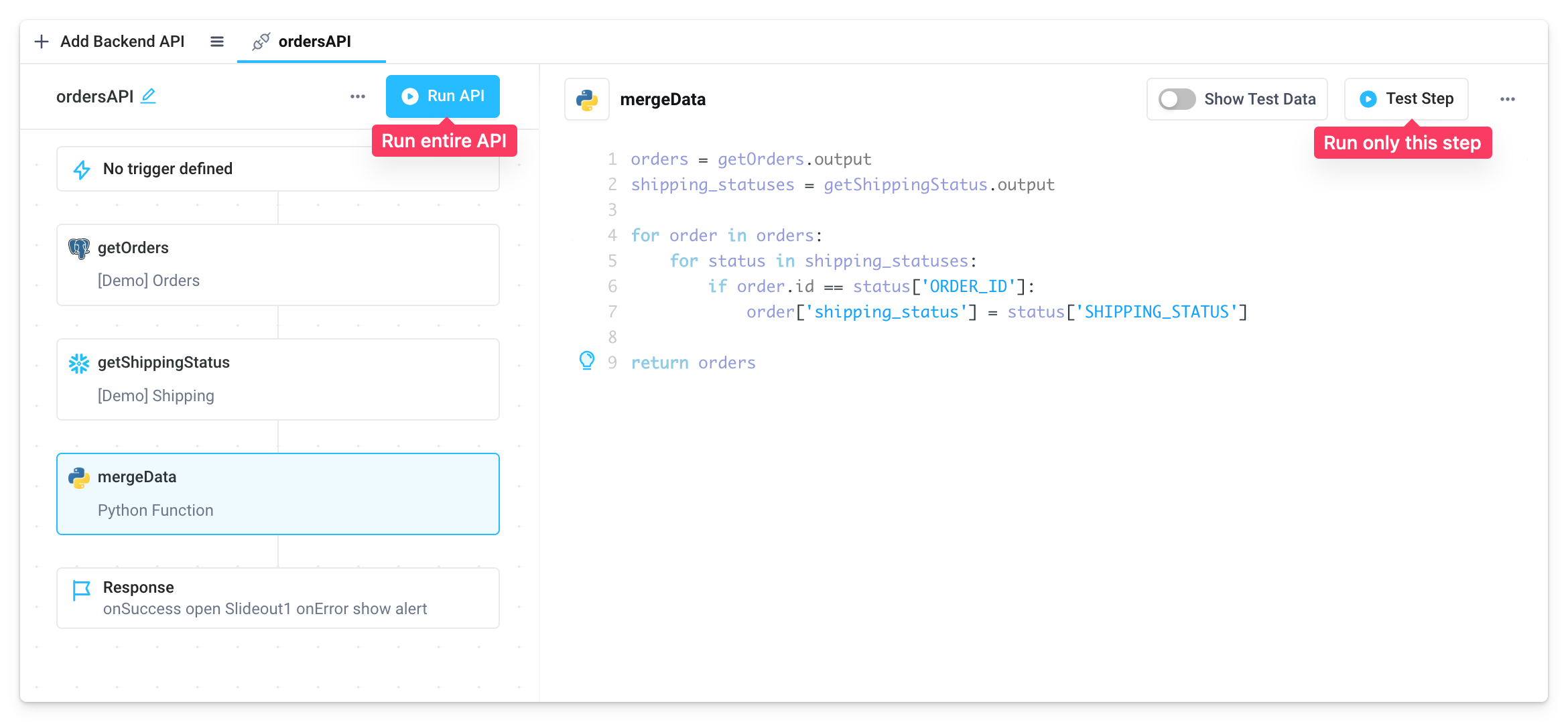
When executing a single step, dependencies on previous steps can be populated based on existing output or overridden by editing the Test Data directly.
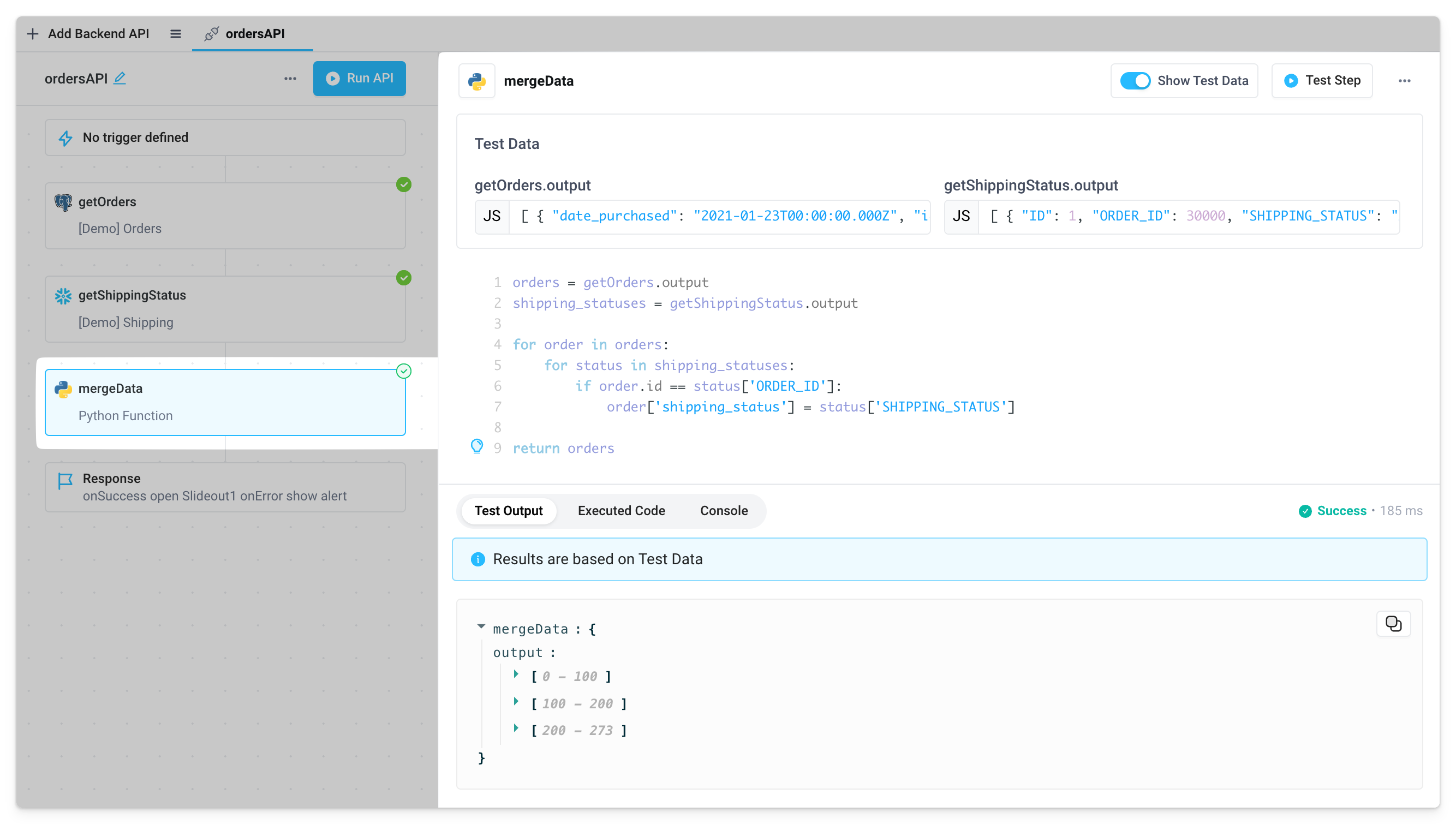
Test Step is also available when building Workflows and Scheduled Jobs.
Using an API response to fill components
The response of a standard synchronous API is the result of the final step in the sequence. You can use the response in the Table Data, Chart Data, Dropdown Data, Grid Data and other related data entry fields in frontend components.
{{API1.response}} // Reference the response of an API in frontend bindings
If you want to access the output of intermediate steps, follow this guide.
In the following example, a frontend Table component is populated with data from a backend API called getOrders
. Note that the API response object must be wrapped in bindings:
Running an API on component event handler
Frontend components have event handlers that can be used to respond to user events. Among other things, they can be used to execute an API based when an event happens. In the following example, the event handler onTextChanged for Inputs triggers a Run API Action Type that runs a backend API called API1
:
API trigger block
The API Trigger block allows you to set when an API is executed and who is allowed to execute this API.
API Triggers
Each backend API has a trigger block that lists which API Triggers cause the API to run.
Run on page load
Learn how you can use a Backend API's on PageLoad event handler to run JavaScript on the frontend of your application when a page loads.
Whether the API also Runs on Page Load event can be set directly in the block:
Always
means the API will be executed on every Page Load eventNever
means the API will never be executed when a Page Load event occursAutomatic
means the backend API will be executed on Page Load whenever there is a visible frontend component that references the response of the API. If this is not the case, the API will not be executed on Page Load.
Example
In the example below Run on page load is set to Automatic
and Superblocks shows that the API API1
is executed Automatic (Currently Will Run) when a page load event occurs.
The reason for this is that there is a table Table1
in the frontend which references the API's response with {{API1.response}}
. As such, Superblocks defaults to running the API automatically so that the table is populated when the page is loaded.
If no frontend component references the API's response, Superblocks displays Automatic (Currently Won't Run) instead.
Inputs and Permissions
The Inputs section lists all frontend components that provide input values to the API. These are snapshotted at API execution time and passed to the API.
Under Permissions you can define who is authorized to execute the backend API. This can either be everyone who has access to the application or only certain users and groups.
API response block
The API response block allows you to control the API Response type, set Frontend Event Handlers, and see where the API is Referenced by components.
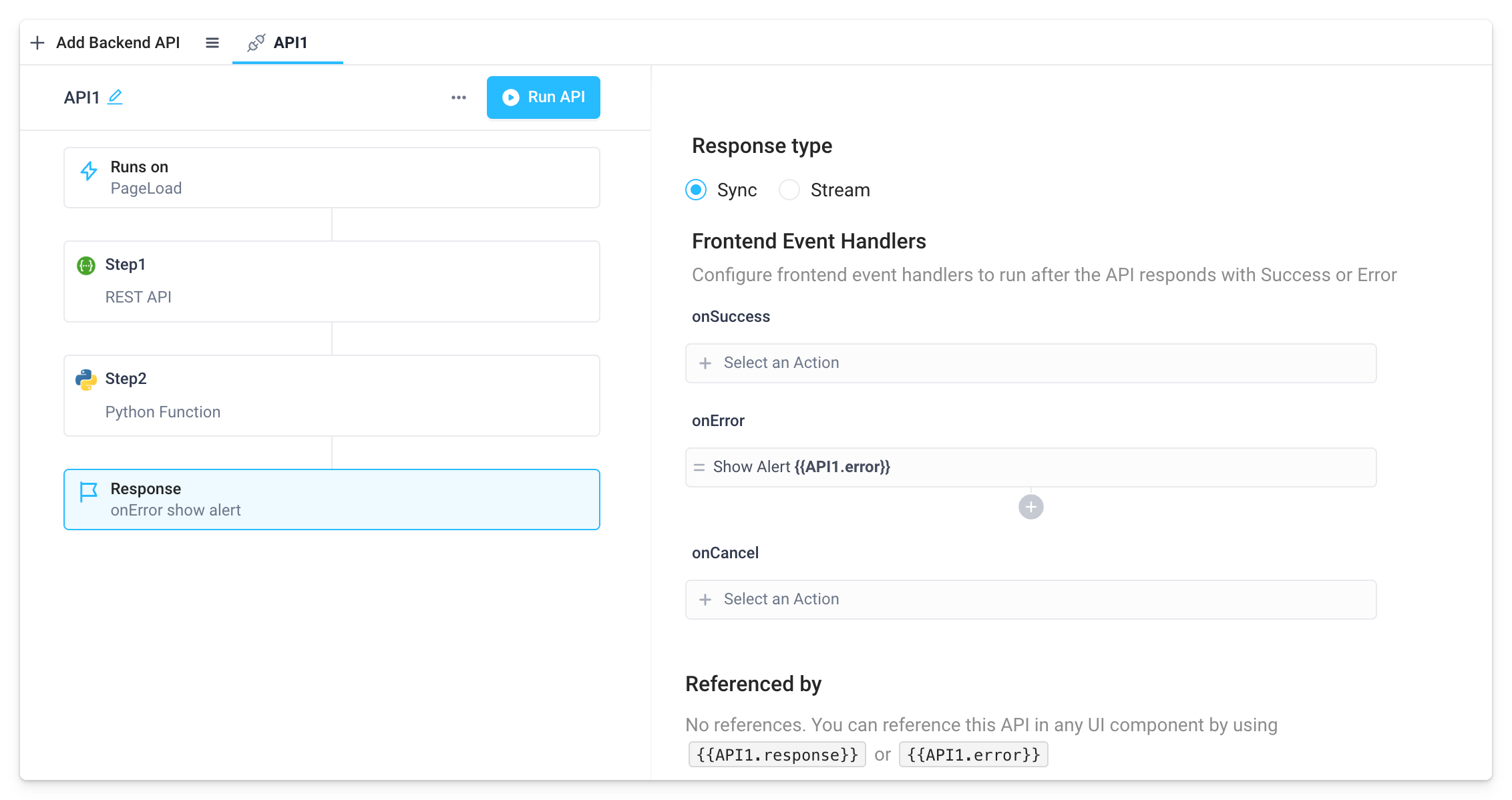
Response type
By default, APIs exchange and process data in a synchronous request-response manner (response type Sync). As such, the output of a synchronous API can be referenced with the API_NAME.response
variable. Most use cases in Superblocks will use this standard response type.
Alternatively, APIs can be configured to send data to the frontend in a real-time continuous stream when using compatible streaming integrations and block types (response type Stream). These APIs do not have a response variable for referencing their output. Read more on how to use streaming APIs in Streaming Applications.
Frontend Event Handlers
Frontend Event Handlers allow you to define frontend actions that are performed based on the backend API results:
Frontend Event Handler | Response types | Called when |
---|---|---|
onSuccess | Sync / Stream | API completes sucessfully |
onError | Sync / Stream | API encounters an error during its execution |
onCancel | Sync / Stream | API is canceled |
onMessage | Stream | Streaming API sends a message |
These event handlers run on the frontend once the backend finishes executing the API (onSuccess
, onError
, onCancel
) or whenever a streaming API processes and sends a message (onMessage
). Therefore, they have access to only the application's frontend scope and to the final data returned / latest value streamed by the API. They do not have access to the output of intermediate API steps, such as Step1.output
.
The onSuccess
and onError
event handlers can also be set in the properties panel at the component level.
onSuccess
When a backend API runs through successfully without throwing any errors, a success event is returned to the frontend, and the onSuccess
event handler is called. The event handler can trigger any number of available action types. The onSuccess
event handler is not set by default.
onError
When an API step encounters an error during execution, that API step will fail. The remaining steps will not run and the output of any failed step will be an empty object.
When a backend API fails, an error event is returned to the frontend, and the onError
event handler is called. The event handler can trigger any number of available action types. By default, the onError
event handler will trigger the showAlert()
action type that displays the error message in the UI.
Additionally, all API step errors will be available to view in the audit logs.
onCancel
When a backend API is canceled by a frontend event handler using the Cancel APIs action type, the onCancel
event handler is called.
onMessage
When a streaming API sends a message to the frontend via the Stream or Send block, the onMessage
event handler is called. This is most useful for updating a frontend frontend variable that is bound to a component for displaying the live streamed data. Read more on how to use the onMessage
event handler in Streaming Applications.
Referenced by
The Referenced by section lists all frontend components that reference a backend API's response. In the following example, backend API AP1
is referenced by a Table component Table1
in the frontend:
Custom response notification example
In this example, we will set up an API to provide custom messages to the end user when the API runs successfully or runs into an error.
On successful execution of the API, the end user will see a message confirming the data was fetched as expected.
When the API encounters an error, the end user will see the error message along with a custom error message indicting next steps on how to resolve the issue.
Duplicating APIs
To copy all the steps within an API to a new API, click Duplicate on the API in the Backend API builder or the Navigation panel.
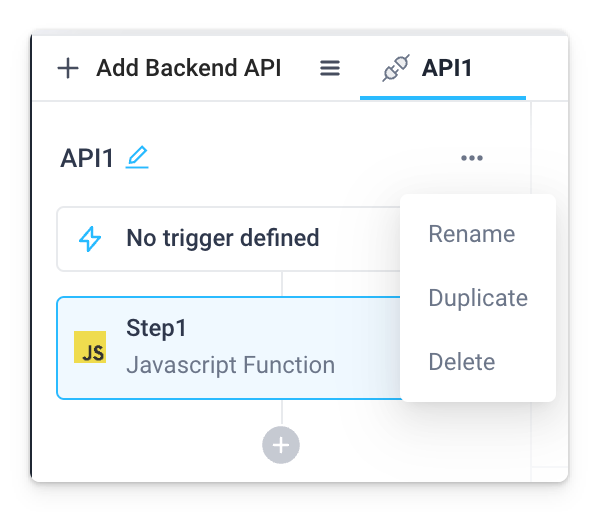
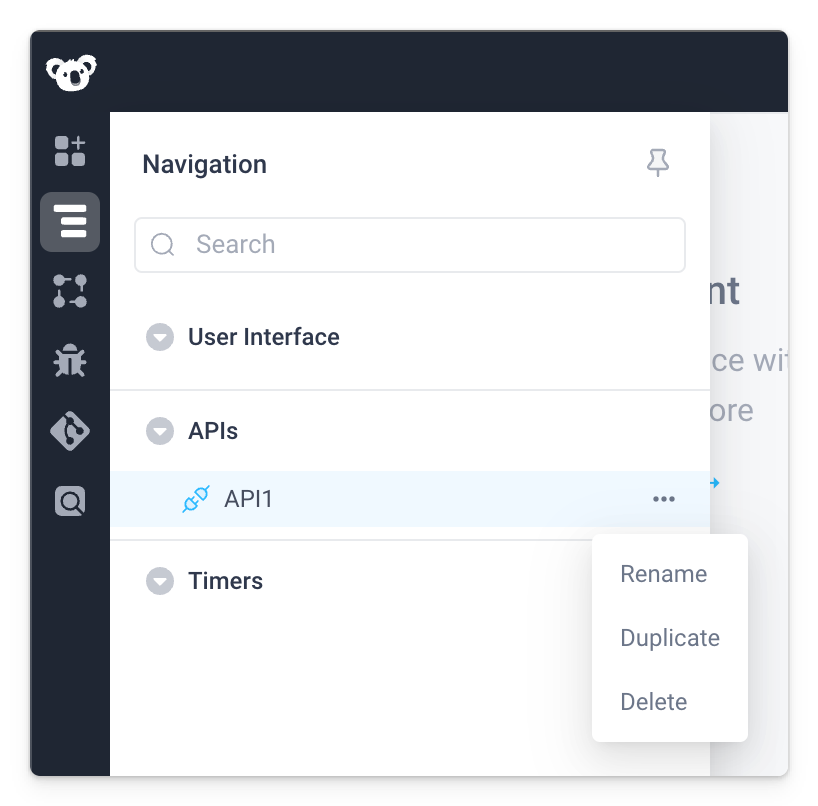
If you find yourself duplicating an API frequently with minimal alterations, consider using Workflows.