Frontend JavaScript
Browser based JavaScript can be written on the frontend directly inside Application UI components:
- Disable/enable a button based on data entered into a form
- Customize a grid's layout with a switch statement
- Conditionally format color of table cells
Bindings
Bindings allow you to insert dynamic data into your components.
Anything inside of {{}}
is evaluated as a JavaScript expression
For example, in a Text widget, we can write: Hi from {{"Superblocks"}}!
. The Text widget is then evaluated to Hi from Superblocks!
When using bindings anywhere in Superblocks, you can reference the built-in JavaScript Array methods available in the autocomplete.
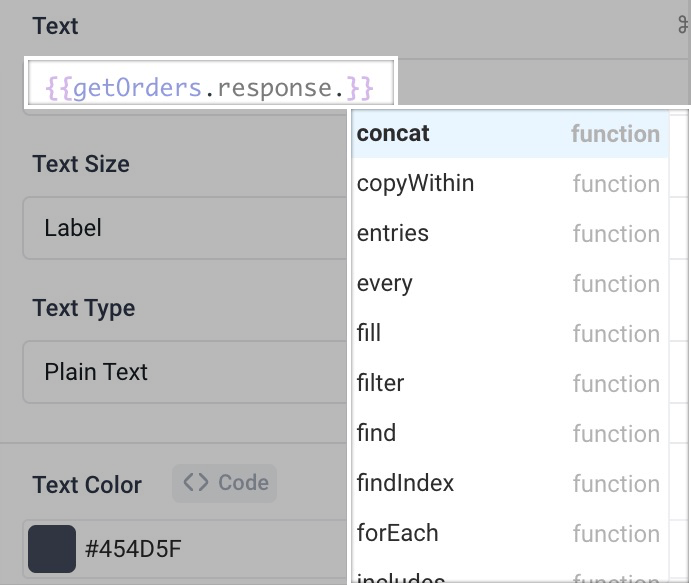
Pop Out Editor
The Pop Out Editor allows you to click a button inside a component property's text block to expand the editor into a tab in the bottom panel. This gives you more room to write code for things like Run JS triggers.
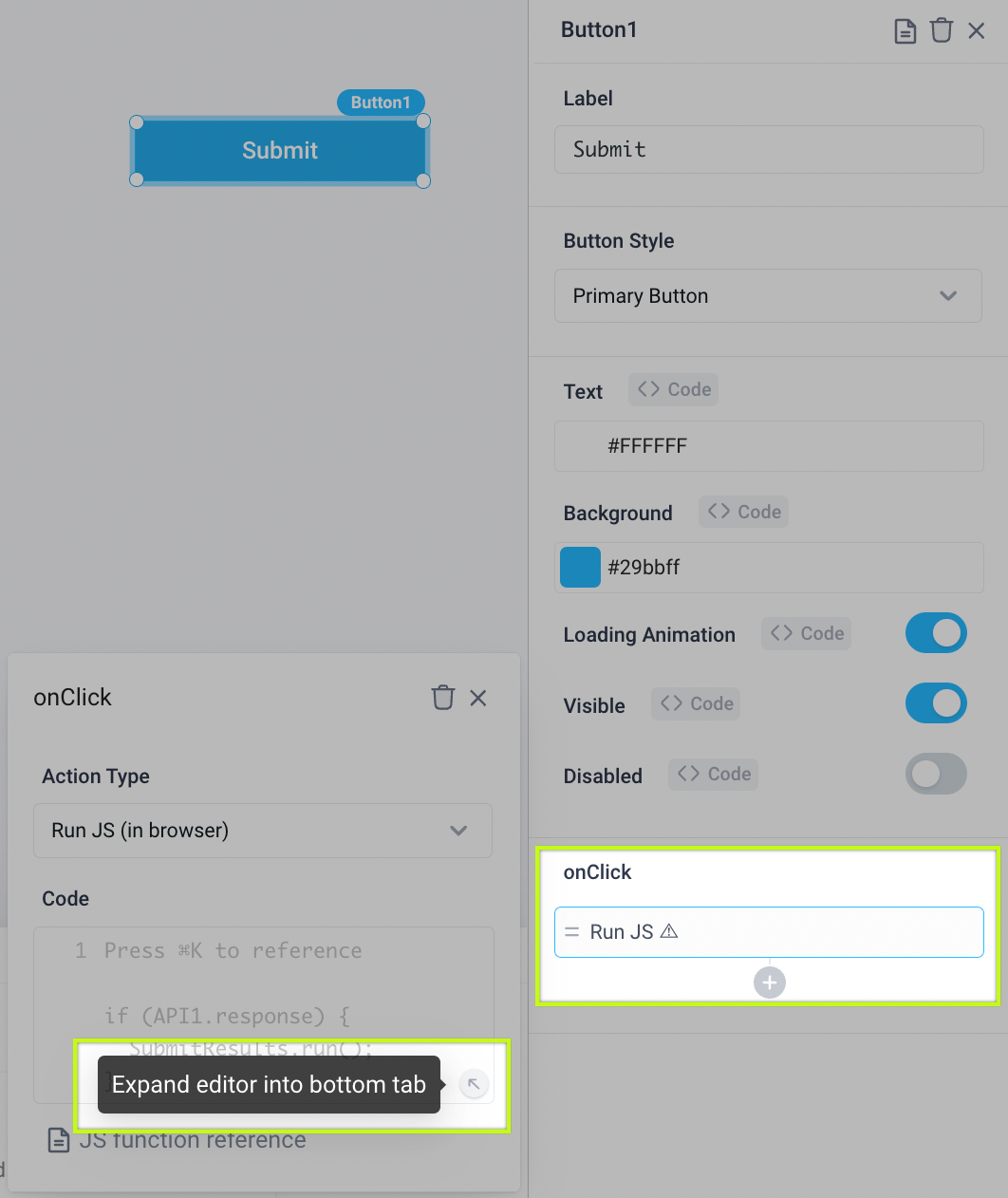
Once popped out, you can adjust the height of the bottom panel to further increase the space for writing code.
Working with Complex JavaScript in Bindings
There are cases where you may want to write more complex JavaScript inside of bindings (i.e. inserting JavaScript that is multiple lines long). To do this, you have to convert your JavaScript into a valid expression. For example, let's say you have a switch statement that you want to use as a binding:
switch (Dropdown1.selectedOptionValue) {
case "NEW_YORK":
return "New York";
case "TORONTO":
return "Toronto";
default:
return "No city defined";
}
We can turn this code into a valid binding by wrapping this code inside of a anonymous function and executing it immediately:
{{(function () {
switch (Dropdown1.selectedOptionValue) {
case "NEW_YORK":
return "New York";
case "TORONTO":
return "Toronto";
default:
return "No city defined";
}
})()
}}
You can use this technique to write any arbitrary JavaScript code inside of bindings!
{{(function () {
<your_javascript_code_here>
})()
}}
Disable/Enable a button based on data entered into a form
Full code snippet below
{{(function() {
if (DatePicker1.selectedDate && Input1.value && Dropdown1.selectedOptionValue) {
return false;
} else {
return true;
}
})()}}
Customize grid based on a switch statement
Full code snippet below
{{(function() {
if (currentCell.dateOfBirth === "") {
return "<b>DOB</b>: <i>Unknown</i>";
} else {
return "<b>DOB</b>: "+currentCell.dateOfBirth;
}
})()}}
Full code snippet below
{{(function() {
switch (currentCell.house) {
case "Gryffindor":
return "#ae0001";
case "Slytherin":
return "#2a623d";
case "Ravenclaw":
return "#000A90";
case "Hufflepuff":
return "#FFDB00";
default:
return "black";
}
})()}}
Conditionally format color of table cells
Full code snippet below
{{currentRow.price > 200 ? "green" : "yellow"}}
Referencing Components
You can refer to other components by using the exact same syntax. For example, let's refer to a Table's currently selected row inside of my Text widget:
Here, we are referring to Table1's selected row and referring to the "city" column of the table by doing {{Table1.selectedRow.city}}
When to Set Local Variables with Run JS Instead of Set Frontend Variable
Even though the optimal method for updating global variables involves using the form-based 'set frontend variable' approach, there may be situations where you have to update a variable on the frontend after a backend API successfully completes its execution, or when an event handler is triggered. These situations arise when we need some logic on the frontend in order to know when or what to set the frontend variable.
When executing frontend JavaScript, the function for setting variables is limited to the Run JS property of events using VARIABLE_NAME.set().
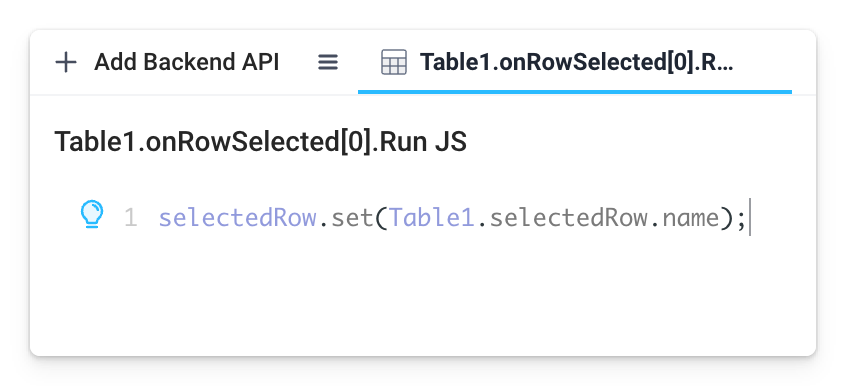
Local Variable Consistency and Avoiding Race Conditions
There are important considerations when using frontend variables to avoid retrieving stale values. Please note that variables are only set with updated values after the entire Run JS block has completed executing. We typically set up additional actions with new Run JS blocks so we can access variables set in previous blocks.
Where possible, it is recommended to use the form based event handlers instead of writing these functions manually with JavaScript. Use these functions only when more complex conditional logic is required.
Here is an example that also uses the same variable set above in our previous example:
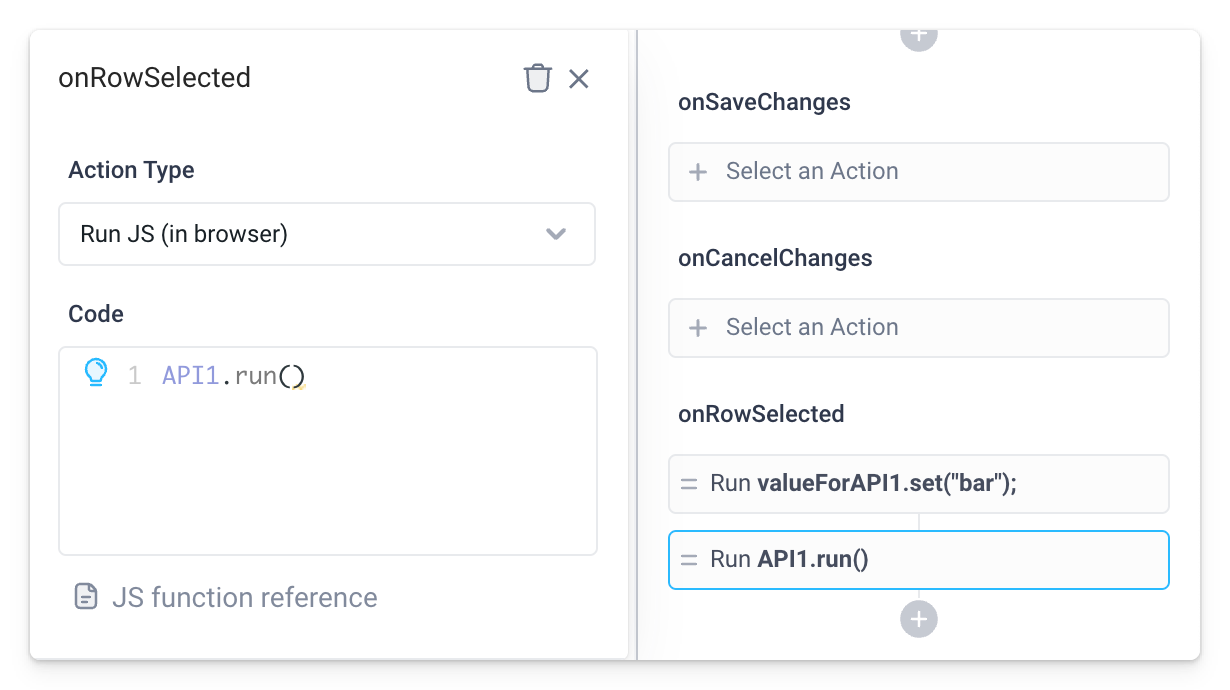
This example will give us unexpected behavior because we're calling an API to use a value that was set in the same block:
