Functions in Run JS
The following built-in JavaScript functions are available in a Run JS Action Type that is triggered by an event handler.
Where possible, it is recommended to use the form based event handlers instead of writing these functions manually with JavaScript. Use these functions only when more complex conditional logic is required.
navigateTo()
This function allows the user to navigate to another URL when triggered. This functionality can also be triggered as its own form-based Action Type from an event handler.
navigateTo(URL, params, target)
Return Type: void
Parameters
Parameter Name | Type | Description | Default |
---|---|---|---|
URL | String | The URL to navigate to. You can also provide a path within the application, such as /detail-page/{{Table1.selectedRow.userId}} , to navigate without reloading the page. | None |
params (optional) | Dictionary | Query parameters, used to pass context to the destination page. | None |
target (optional) | String | "SAME_WINDOW" or "NEW_WINDOW", case-sensitive. Defines whether the page opens in the same or a new window. | SAME_WINDOW |
Passing in key:value pairs can be omitted by using empty brackets {}
allowing the target to be specified. E.g. navigateTo("URL", {}, "NEW_WINDOW")
Example
navigateTo("https://superblocks.com",{"user_id": 123, "email": "user@company_example.com"},"NEW_WINDOW");
If navigateTo()
links to another Superblocks Application, the query parameters passed can be accessed with Global.URL.queryParams.<query_parameter_key>
. (Learn more about the Global object metadata)
This gif shows an example of navigating to another application when a row is selected:
showAlert()
This functions shows an alert on trigger allowing you to specify the message and style of the alert. This functionality can also be triggered as its own form-based Action Type from an event handler.
showAlert(alert_message, style, durationSeconds, position)
Return Type: void
Parameters
Parameter Name | Type | Description | Default |
---|---|---|---|
alert_message | String | Message to be shown to the user. | None |
style (optional) | String | "success", "info", "warning", "error", case-sensitive. The alert message style. | info |
durationSeconds (optional) | Number | The duration before the alert is automatically dismissed. Specifying 0 will make the alert sticky to require explicit dismissal. | 4 seconds |
position (optional) | String | “bottomRight”, “bottom”, “bottomLeft”, “topRight”, “top”, “topLeft” | bottomRight |
Example
showAlert("Woohoo, it was a success!" ,"success", 10, "bottomRight")
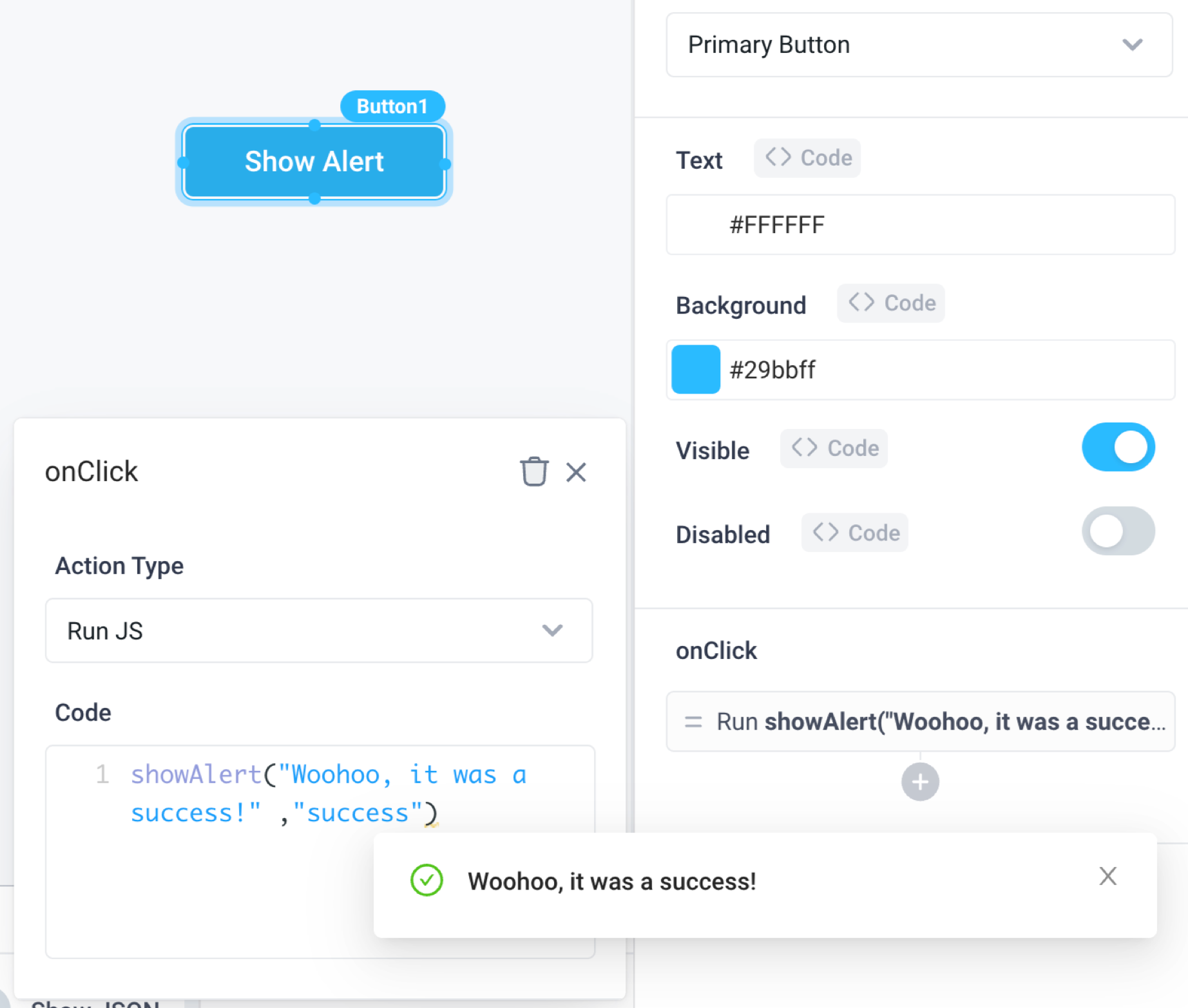
storeValue() - Deprecated
StoreValue is deprecated. Please use Frontend Variables to store values in local browser storage.
This functions allows you to store a value in the local browser storage, similar to a frontend variable, then access it via the Global object.
storeValue(key, value, isTemporary)
Return Type: void
Use Global.store.key
to access the value in another component or API step.
Parameters
Parameter Name | Type | Description | Default |
---|---|---|---|
key | String | A string containing the name of the key. | None |
value | String | Value stored in the provided key. Set to undefined to delete the variable from local storage. | None |
isTemporary (optional) | Boolean | Controls the persistence of the local store. Defaults to false so that data is available across sessions. Set to true for a temporary local store that is cleared when the page is refreshed. | false |
Example
- Set and call a local variable
- Set the variable from an Input component with
storeValue("animal",Input1.value)
- Access the stored value using
{{Global.store.animal}}
- Clear the value by passing in an empty string as the value. E.g.
storeValue("animal","")
- Set the variable from an Input component with
- Delete a local variable
- Set the value of a variable to
undefined
to delete it. E.g.{{storeValue("animal", undefined)}}
- Here is an example script to delete all local variables:
- Set the value of a variable to
(function() {
var store_object = Global.store;
var store_keys_array = Object.keys(store_object);
for (let i in store_keys_array) {
storeValue(store_keys_array[i], undefined);
}
})();
copyToClipboard()
This function allows you to copy text to the clipboard.
copyToClipboard(message)
Return Type: void
Parameters
Parameter Name | Type | Description | Default |
---|---|---|---|
message | String | A string to be copied to clipboard. | None |
Example
copyToClipboard(Button1.text)
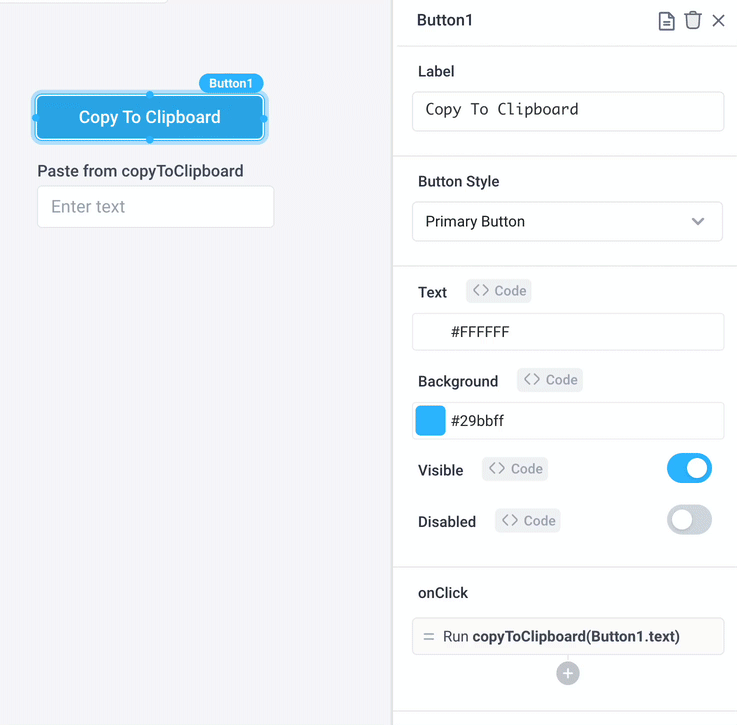
resetComponent()
This functions resets a component to its default state. This functionality can also be triggered as its own form-based Action Type from an event handler.
resetComponent(component, resetChildren)
Return Type: void
Parameters
Parameter Name | Type | Description | Default |
---|---|---|---|
component | String | Components whose properties are to be reset to their default state. | None |
resetChildren (optional) | Boolean | Reset all child components in addition to the component itself. | false |
Example
resetComponent('Input1')
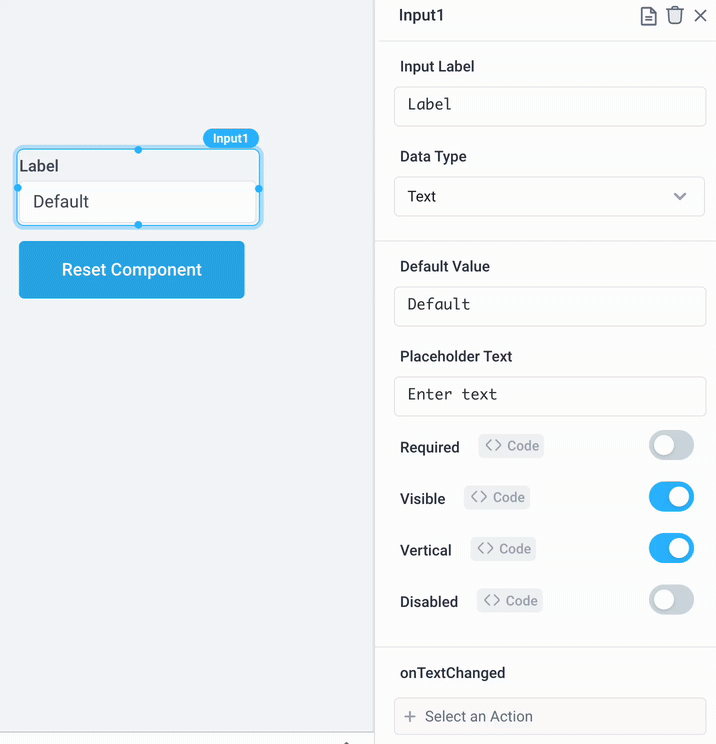
When resetting a Container component, you can choose to reset all children components within that container using resetChildren
.
See also resetting components from event handlers.
component.set()
This function sets the property of a component to a specified value. This functionality can also be triggered as its own form-based Action Type from an event handler.
Visit the individual component pages for a list of the settable component properties, for example the Settable properties of the Table component.
component_name.set(propertyName, value)
Return Type: void
Parameters
Parameter Name | Type | Description |
---|---|---|
propertyName | String | Property to set. |
value | Depends on property | Value of property. |
View the full list of properties and example values here.
Example
Dropdown1.set('selectedOptionValue','Boston');
See also setting component properties from event handlers.
logoutIntegrations()
This function clears any cached authentication tokens. This is mostly used for integrations using OAuth where you may need to authenticate using another account than the one that the integration was set up with.
logoutIntegrations()
Return Type: void
Example
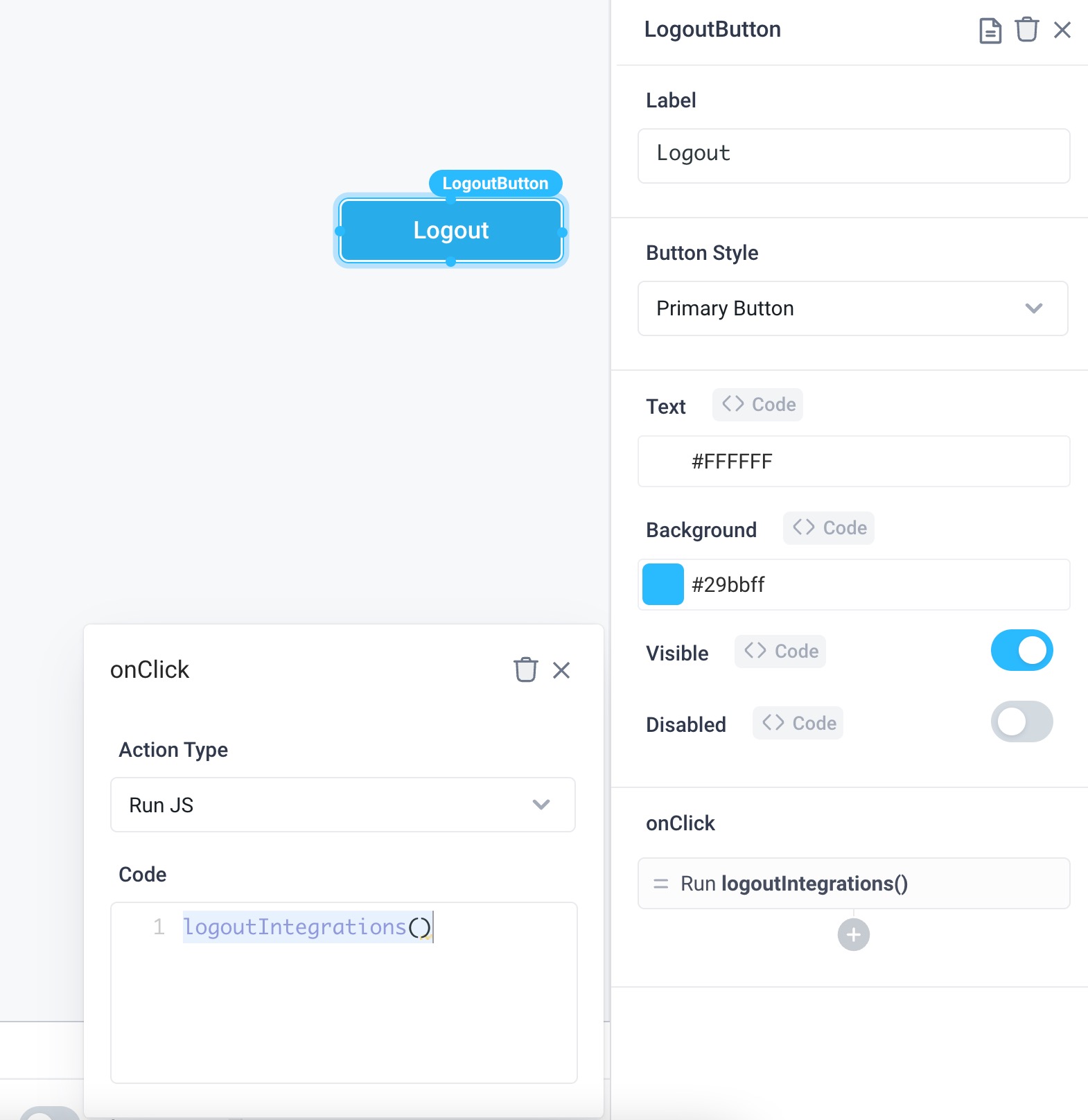
download()
This function allows you to trigger a file download from frontend JavaScript.
download(data, fileName, fileType)
Return Type: void
Parameters
Parameter Name | Type | Description | Default |
---|---|---|---|
data | String | Data or URL to be saved locally. | None |
fileName | String | File name to be saved locally. | None |
fileType | String | MIME type of the file to be saved locally. | text/plain |
Examples
The download()
function uses downloadjs under the hood. Reference those docs for additional examples.
Plain text string
download("This is my text.", "myfile", "text/plain");
Image from data URL
download("data:image/jpeg;base64,<BASE64_STRING>", "test.jpg", "image/jpeg");
Image from external URL
download("https://upload.wikimedia.org/wikipedia/commons/4/49/Koala_climbing_tree.jpg")
slideout.open()/close()
This function opens or closes a Slideout component. This functionality can also be triggered as its own form-based Action Type from an event handler.
Open a Slideout component:
slideout_name.open()
Return Type: void
Close a Slideout component:
slideout_name.close()
Return Type: void
See also controlling Slideout components from event handlers.
Definitions
Definition | Description |
---|---|
slideout_name | Name of slideout to be opened/closed. |
Example
Slideout1.open()
modal.open()/close()
This function opens or closes a Modal component. This functionality can also be triggered as its own form-based Action Type from an event handler.
Open a Modal component:
modal_name.open()
Return Type: void
Close a Modal component:
modal_name.close()
Return Type: void
See also controlling Modal components from event handlers.
Definitions
Definition | Description |
---|---|
modal_name | Name of modal to be opened/closed. |
Example
Modal1.open()
timer.start()/stop()/toggle()
This functionality can also be triggered as its own form-based Action Type from an event handler.
Start a Timer:
timer_name.start()
Return Type: void
Stop a Timer:
timer_name.stop()
Return Type: void
Toggle a Timer:
timer_name.toggle()
Return Type: void
Toggling a Timer switches it from on to off or vice versa
Definitions
Definition | Description |
---|---|
timer_name | Name of Timer to be started/stopped/toggled. |
Example
To toggle a Timer called Timer1
:
Timer1.toggle()
See also controlling Timers from event handlers.
API.run()
This function allows the user to start an API from the frontend. This functionality can also be triggered as its own form-based Action Type from an event handler.
The run()
function has no return value, and does not wait for the API to complete.
API_name.run()
Return Type: void
Definitions
Definition | Description |
---|---|
API_name | Name of API to be run. |
Example
For an API called API1
, you can call this from a Run JS function by using:
API1.run()
API.clearResponse()
This function allows the user to clear the response of a backend API. Executing this function removes the data from the state of any frontend component that references the API's output.
API_name.clearResponse()
Return Type: void
Definitions
Definition | Description |
---|---|
API_name | Name of API to clear its response. |
Example
For an API called API1
, you can call this from a Run JS function by using:
API1.clearResponse()
API.cancel()
This function allows the user to cancel an API from the frontend. This functionality can also be triggered as its own form-based Action Type from an event handler.
API_name.cancel()
Return Type: void
(The function has no return value, and does not wait for the API to complete.)
Definitions
Definition | Description |
---|---|
API_name | Name of API to be canceled. |
Example
For an API called API1
, you can call this from a Run JS function by using:
API1.cancel()
variable.set()/setProperty()/resetToDefault()
These functions allow you to manipulate the values of Frontend Variables.
variable.set()
This functionality can also be triggered as its own form-based Action Type from an event handler.
Set a Frontend Variable:
variable_name.set(data: any)
Return Type: void
Parameters
Parameter Name | Type | Description | Default |
---|---|---|---|
data | any | Data to be saved in the variable. | None |
variable.setProperty()
Set a Frontend Variable's Property:
variable_name.setProperty(path: string, data: any)
Return Type: void
Parameters
Parameter Name | Type | Description | Default |
---|---|---|---|
path | string | Path to property | None |
data | any | Data to be saved in property. Set to undefined to delete a property. | None |
variable.resetToDefault()
This functionality can also be triggered as its own form-based Action Type from an event handler.
Reset a Frontend Variable to its default value. If the user has not defined a custom default value, value is reset to null
:
variable_name.resetToDefault()
Return Type: void
Definitions
Definition | Description |
---|---|
variable_name | Name of variable to be set/reset |
Example
In the following example it is assumed that the current value of a Frontend Variable is {'name': 'Billie', 'age': 24}
. The line of code sets the name
property to a different value:
variable_name.setProperty("name", "Lisa");
event.trigger()
This functionality can also be triggered as its own form-based Action Type from an event handler.
event_name.trigger(payload: {})
Return Type: void
Parameters
Parameter Name | Type | Description | Default |
---|---|---|---|
payload | Object containing the arguments to pass to the event. | None |
Example
In the following example, the event named Event_submitOrder
takes an order_id
argument. This value of this argument is provided from an Input component.
Event_submitOrder.trigger({'order_id': orderInput.value});