Workflows Overview
A Workflow is an internal tool that executes a set of steps across your systems: APIs, databases, and business logic in code.
What is a Workflow?
Example use cases
- Send a Slack message every time a signup happens in your app, after enriching it with database info, triggered by your code
- Update your reporting database every time a user clicks "Checkout" on your website via a webhook in Segment
- Return geocoding data on a given address and use it as a "Platform API", accessible from other Superblocks Workflows, Applications, or your existing admin tooling
For detailed examples that include steps for calling external or internal APIs, running custom Python functions, and notifying users via email, check out these Workflow Guides.
Workflows are modular, reusable platform APIs that can be used across all your internal Applications, Workflows, and Scheduled Jobs. Consume Workflows like any REST API.
Workflow setup
On the Superblocks Home page, click Create New followed by Create Workflow.
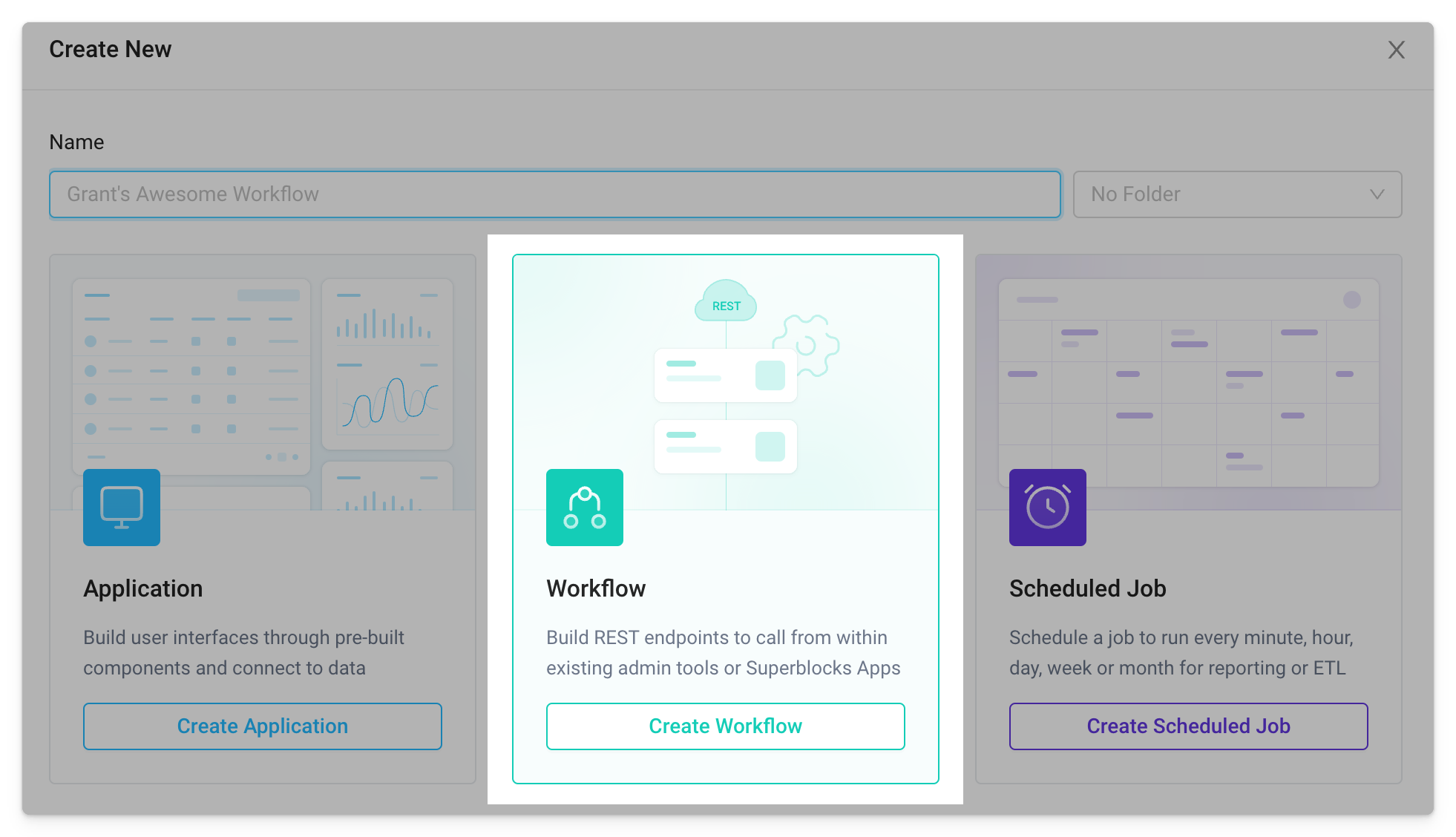
We receive confirmation that the workflow was successfully created and are prompted to setup an optional workflow body.
Workflow body
Workflows can extract request query parameters and body values from the calling request. For example, imagine the service that calls our workflow passes along a ?key
query parameter in the URL and a request_id
in the POST request body. We can reference the values assigned to these in subsequent workflow steps using params.key
and body.request_id
, respectively.
Note, the values defined here are for testing the in-development workflow. In the example below, anytime we test run the workflow, key=value
will be added as a URL query parameter along with "request_id":123
in the request body. By comparison, the deployed workflow will use the actual values contained in the calling request.
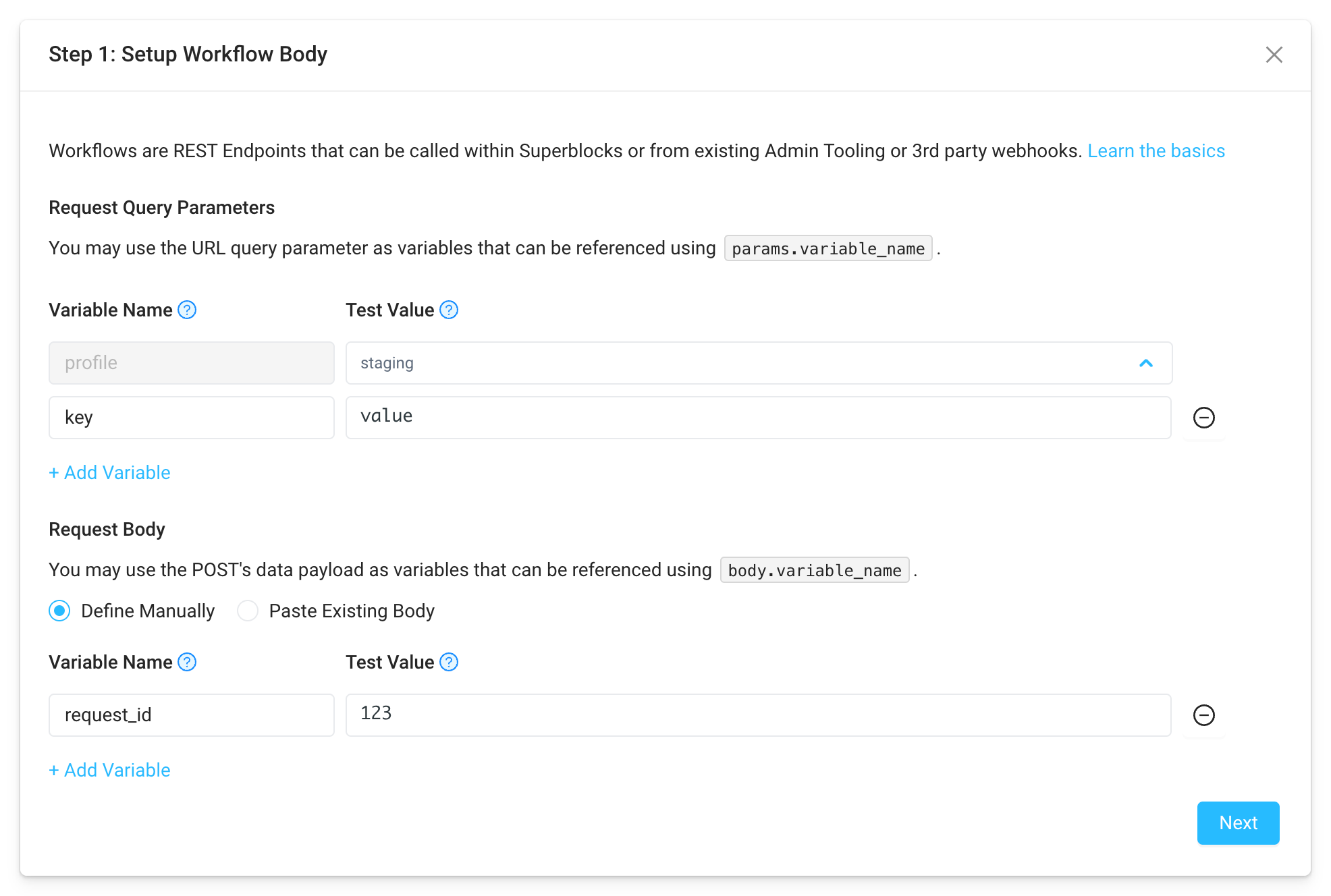
Workflow test
With the workflow URL and test body configured, we can run a test using cURL, Postman, or via code.
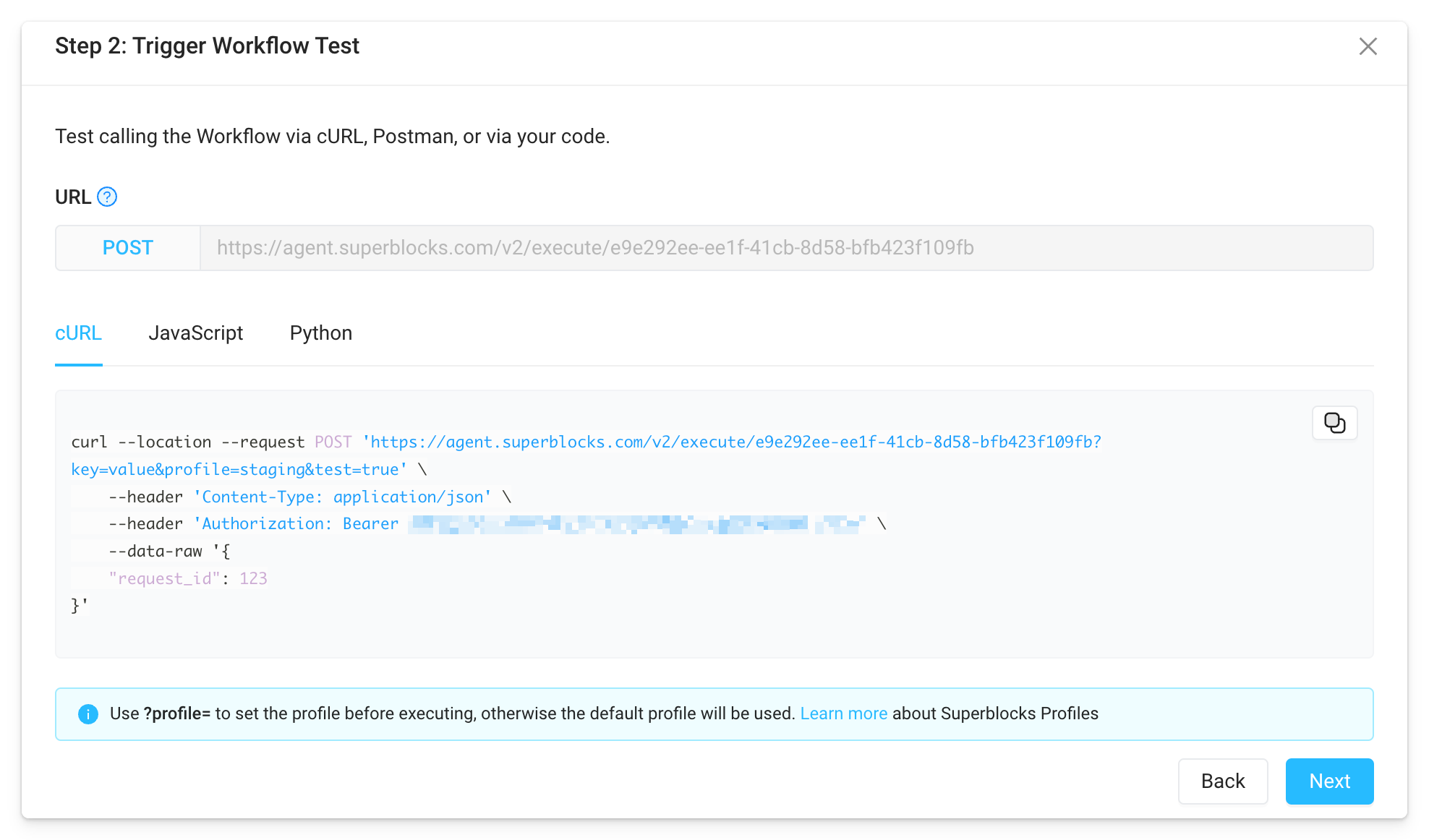
When testing, the query param ?test=true
tells Superblocks to run your in-development workflow. Remove this parameter in order to call your deployed workflow.
In a terminal, execute the curl command above to see that the workflow returns a successful response to the request.
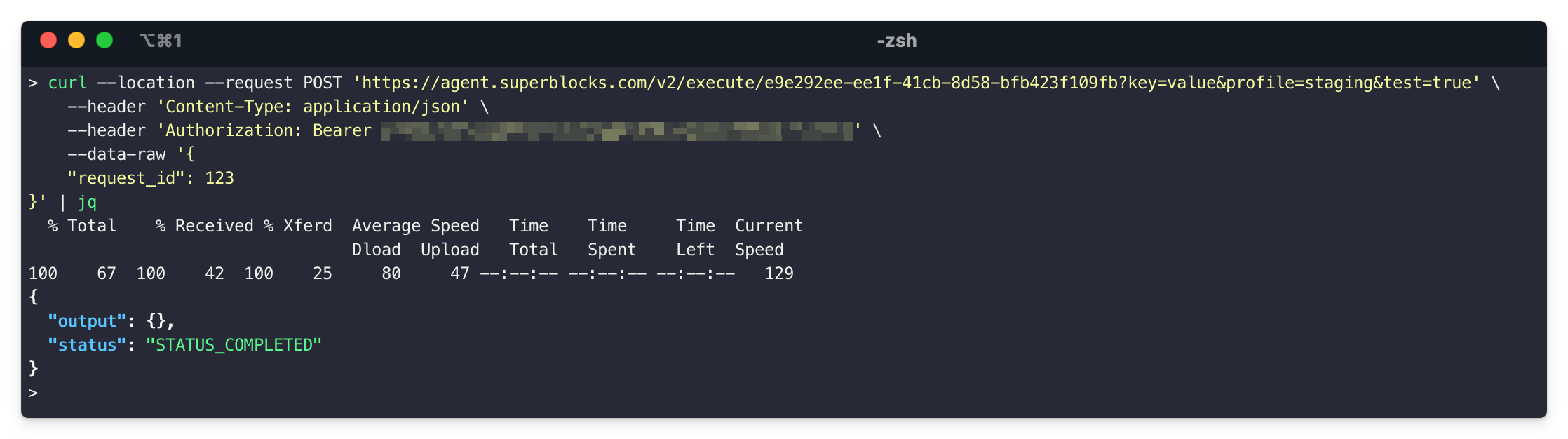
Similarly, we can call the workflow from Postman by importing the curl command there.
Workflow steps
Next, choose from any Superblocks integration to get started adding steps to the workflow.
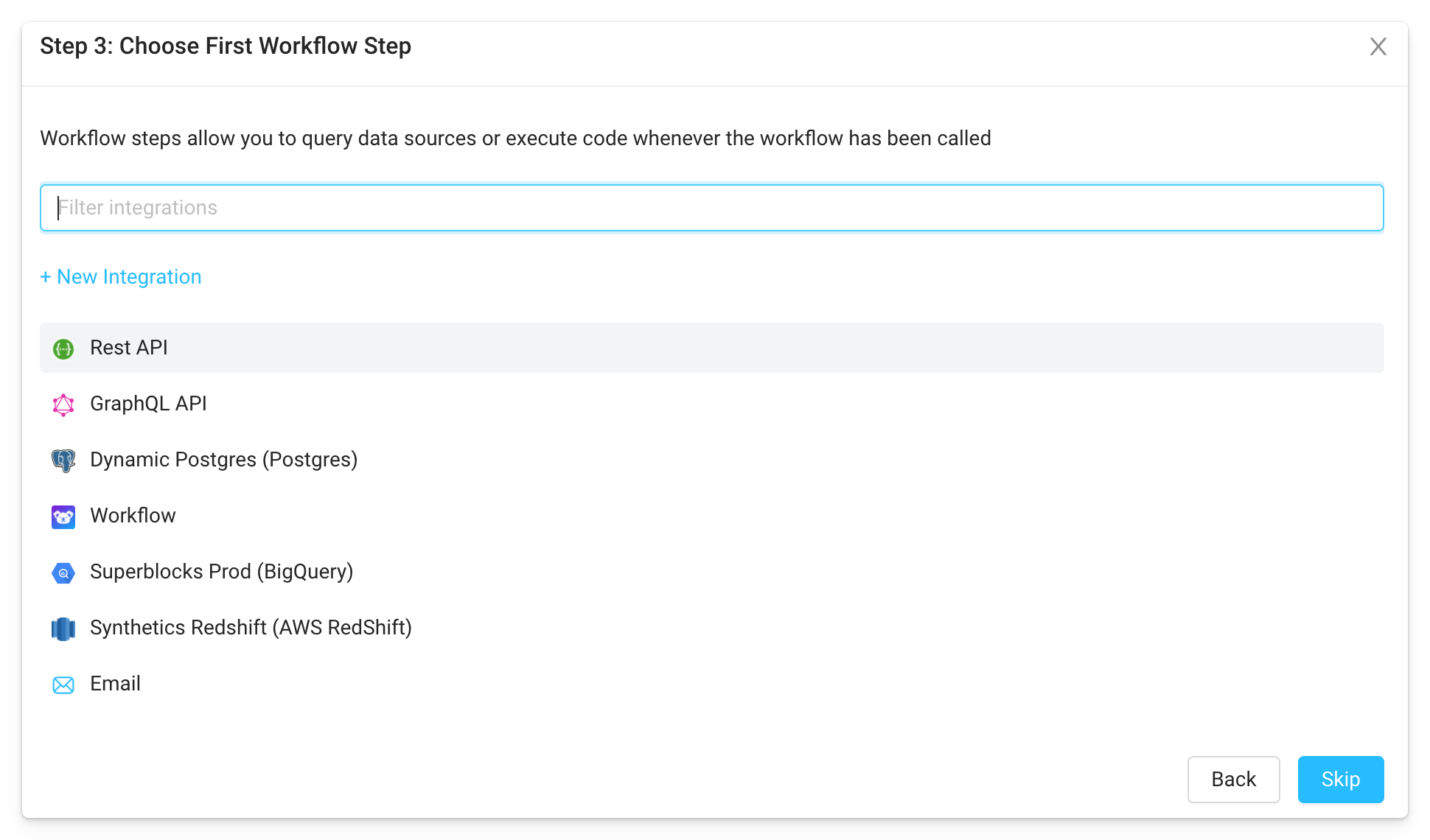
Adding steps in Workflows is exactly the same as defining Superblocks APIs within Applications or Scheduled Jobs.
Example two step Workflow
Let's add steps for the following:
- Call an internal GraphQL API that handles refunds
- Send an email to the user with confirmation of their refund
Since these workflow steps will take an order id and email, let's add those as test variables to the request body in the workflow configuration.
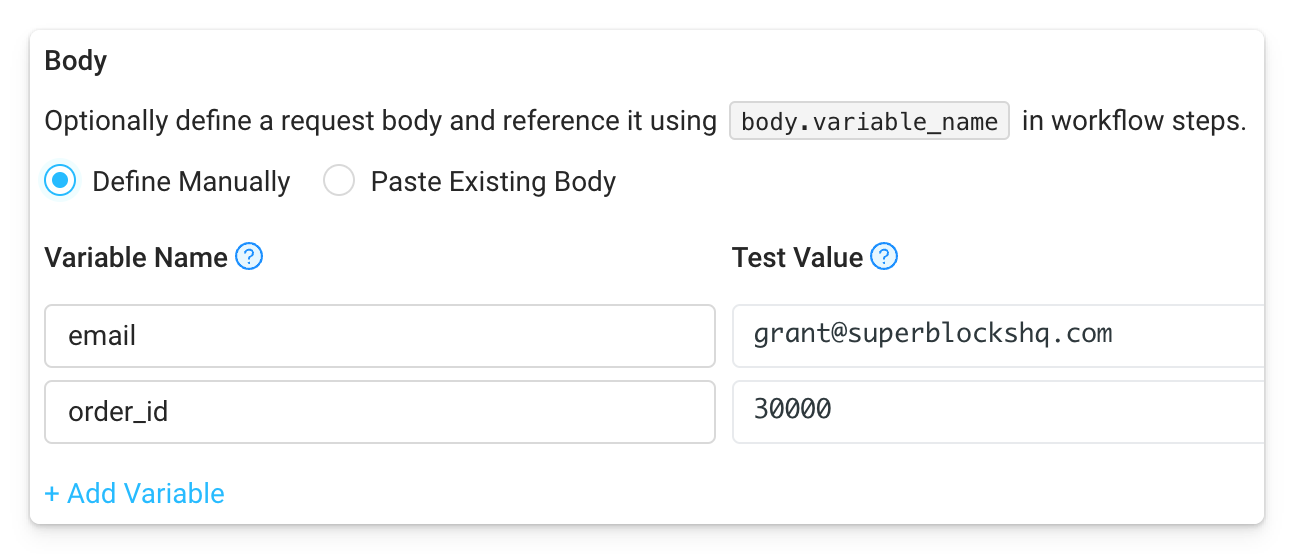
Now we can add the GraphQL API step with the URL and Query for refunding an order, referencing the order id with {{body.order_id}}
. A test run shows the API returns the data for the order id.
With a successful response to our refund API, we'll send the user an email to let them know their order was refunded successfully. The order id is populated in the subject and HTML templated message via {{body.order_id}}
.
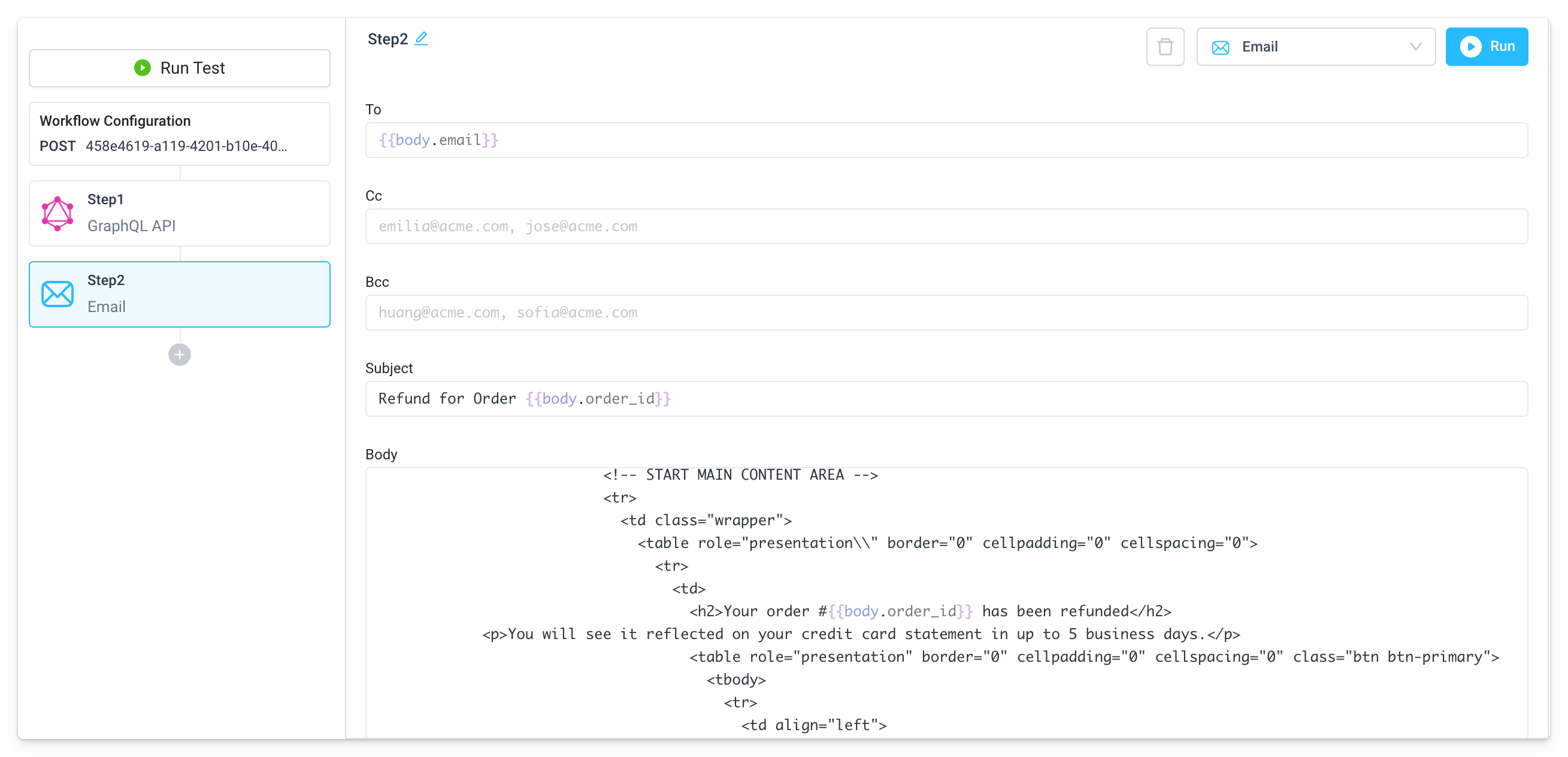
After running a test, the email is received.
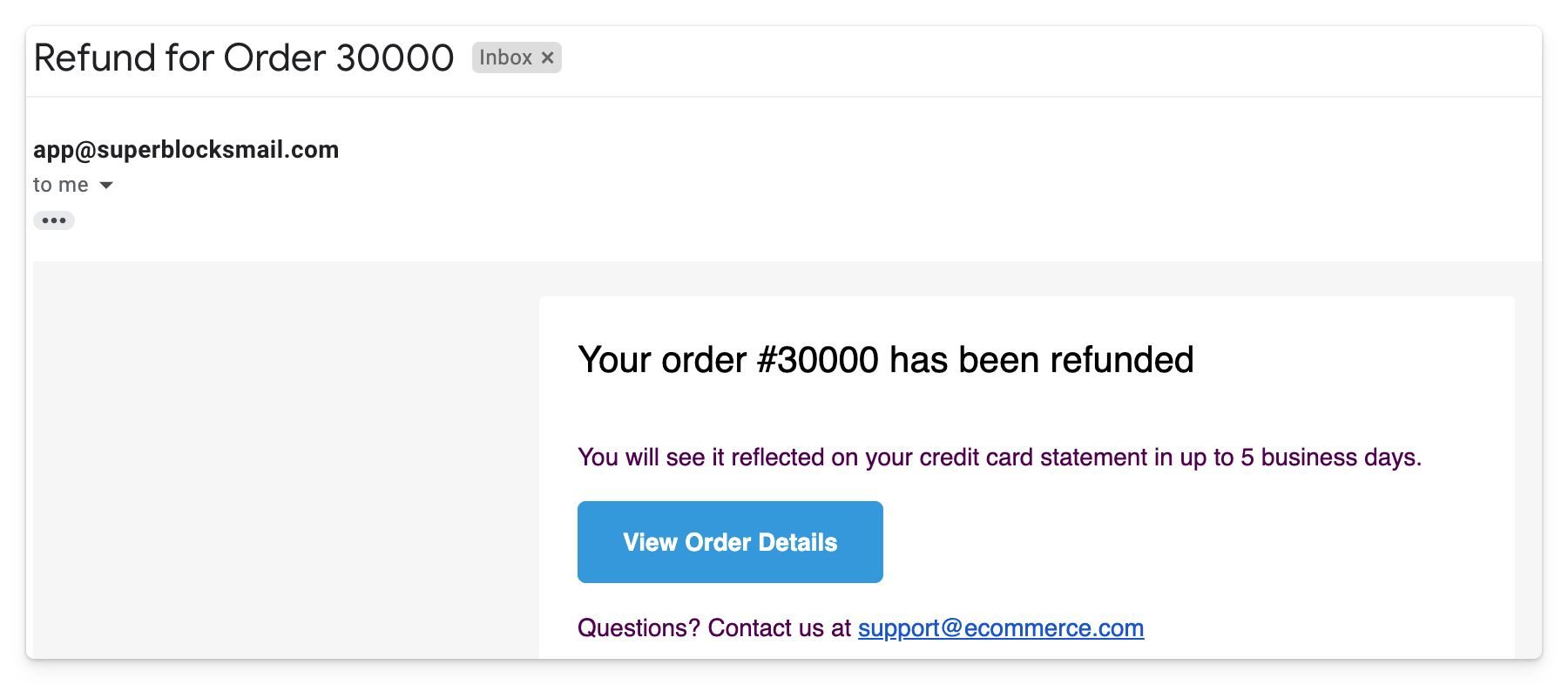
With the workflow steps configured and tested, we can now deploy the workflow.
Workflow deployment
Before running a workflow outside of the development mode, it must first be deployed. To deploy a workflow, click the blue Deploy button.
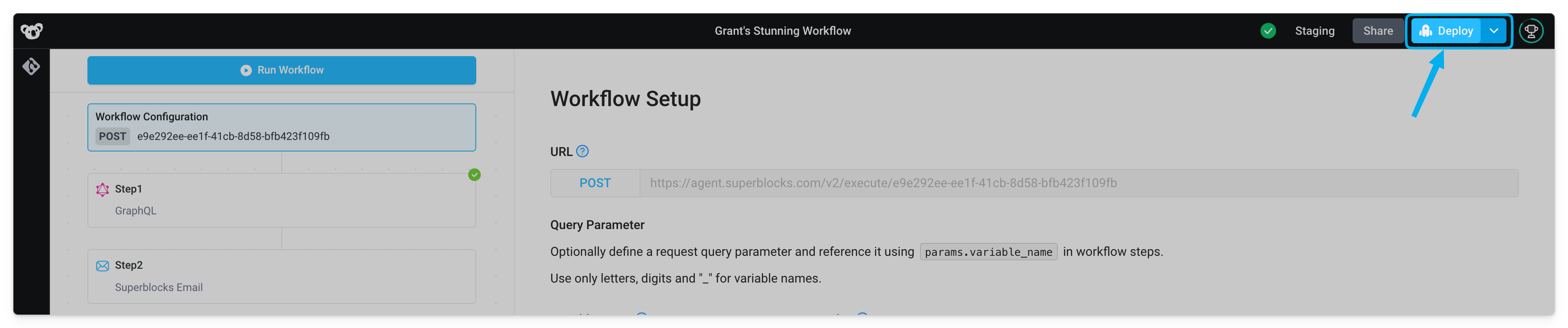
This prompts you to add a commit message and deploy your changes.
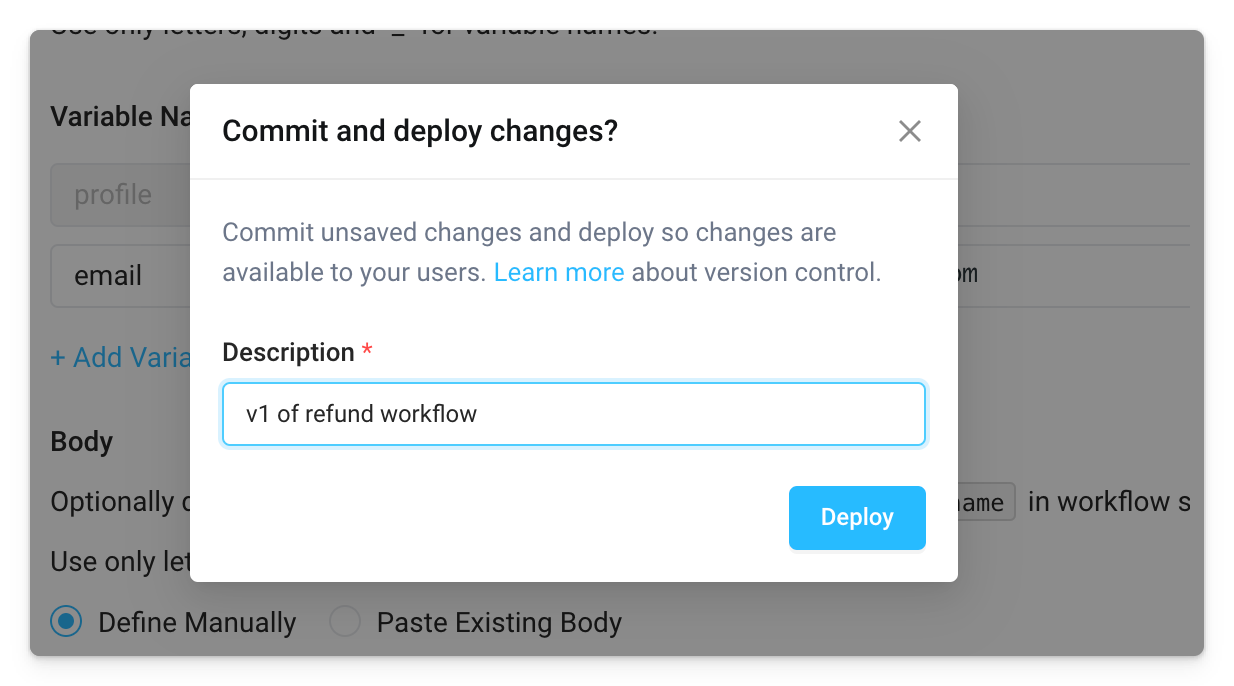
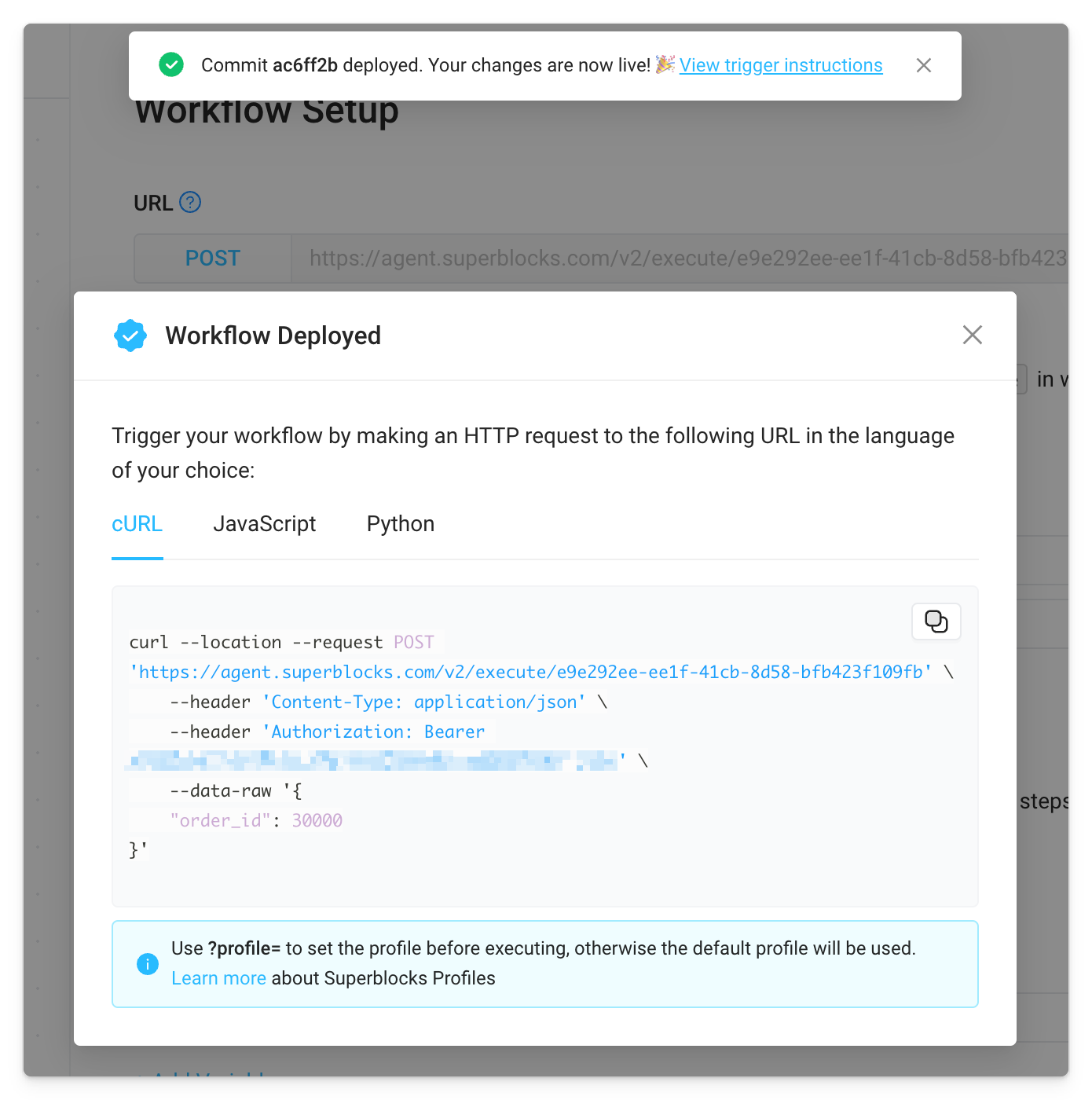
When sending requests to the Workflow, use the query parameter ?profile
to specify which Profile configuration to use when executing. If not specified, the default Profile configured for that Workflow will be used. Learn more about Superblocks Profiles.
After deploying the workflow, it is available externally to 3rd party services or other Superblocks apps.
Run Workflow from 3rd Party
For examples of triggering workflows as webhooks from 3rd parties, check out the Intercom to Zendesk and GitHub to Slack use cases in the Workflow Library.
Workflows are great for automating tasks to connect data from 3rd party systems:
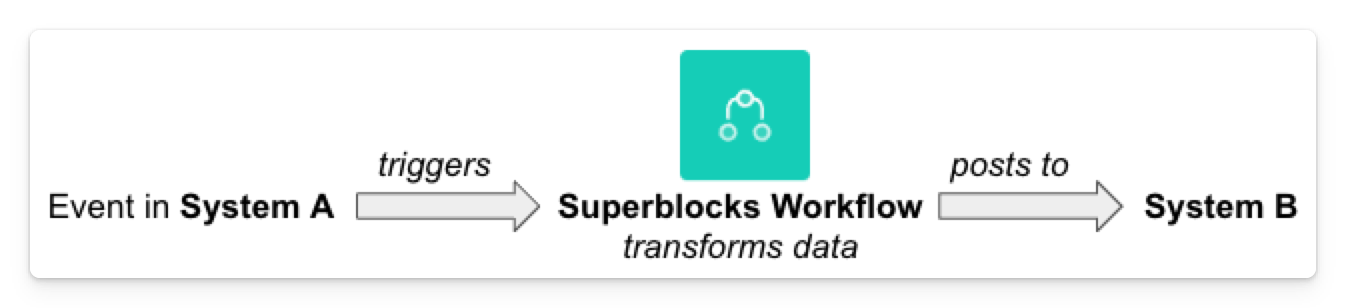
Since a workflow is a REST API, it can be triggered with an HTTP POST request (e.g., a 3rd party webhook or HTTP client libraries in code). A workflow URL follows this structure:
https://agent.superblocks.com/v2/execute/<WORKFLOW-ID>
For example requests, scroll down to the Trigger Workflow section of your workflow. The request requires passing in your account's authorization token using either of these methods:
- As a header:
Authorization: Bearer <TOKEN>
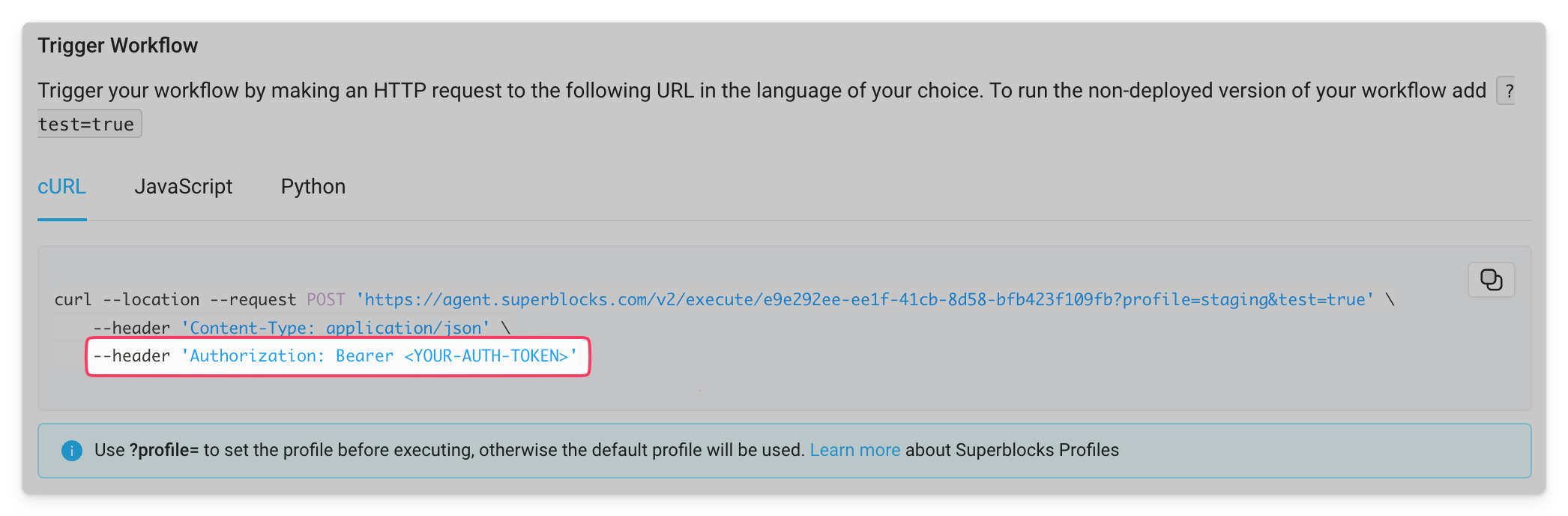
- As a URL query parameter:
sb-auth=<TOKEN>
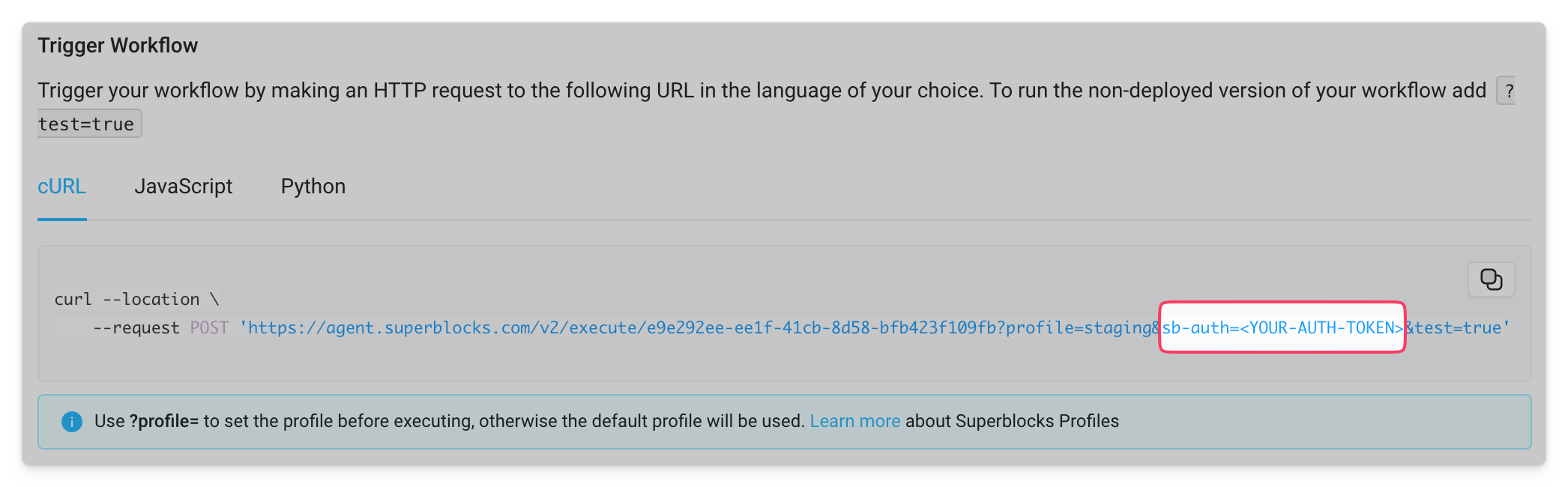
When specifying your auth token as a URL query parameter, be sure to URL encode any special characters.
Async workflows
By default, a client calling a workflow must wait for the workflow to complete before it receives a response.
Alternatively, if you do not need to wait for the response of a workflow, you can trigger it to run asynchronously by adding async=true
as a URL query parameter to the workflow request (For customers on the On-Premise Agent, this is available as of agent v1.2.1).
Run Workflow within Superblocks
If there is a set of common API steps needed in multiple apps, we can create a single workflow and reference that anywhere in Superblocks.
For example, say our teams have multiple apps within Superblocks that all require querying the same database for customer orders, and enriching that data with shipping information from another source. To reuse this logic anywhere, we have created a workflow that takes an order_id
, queries data from Postgres and Snowflake, and returns the merged datasets with Python.
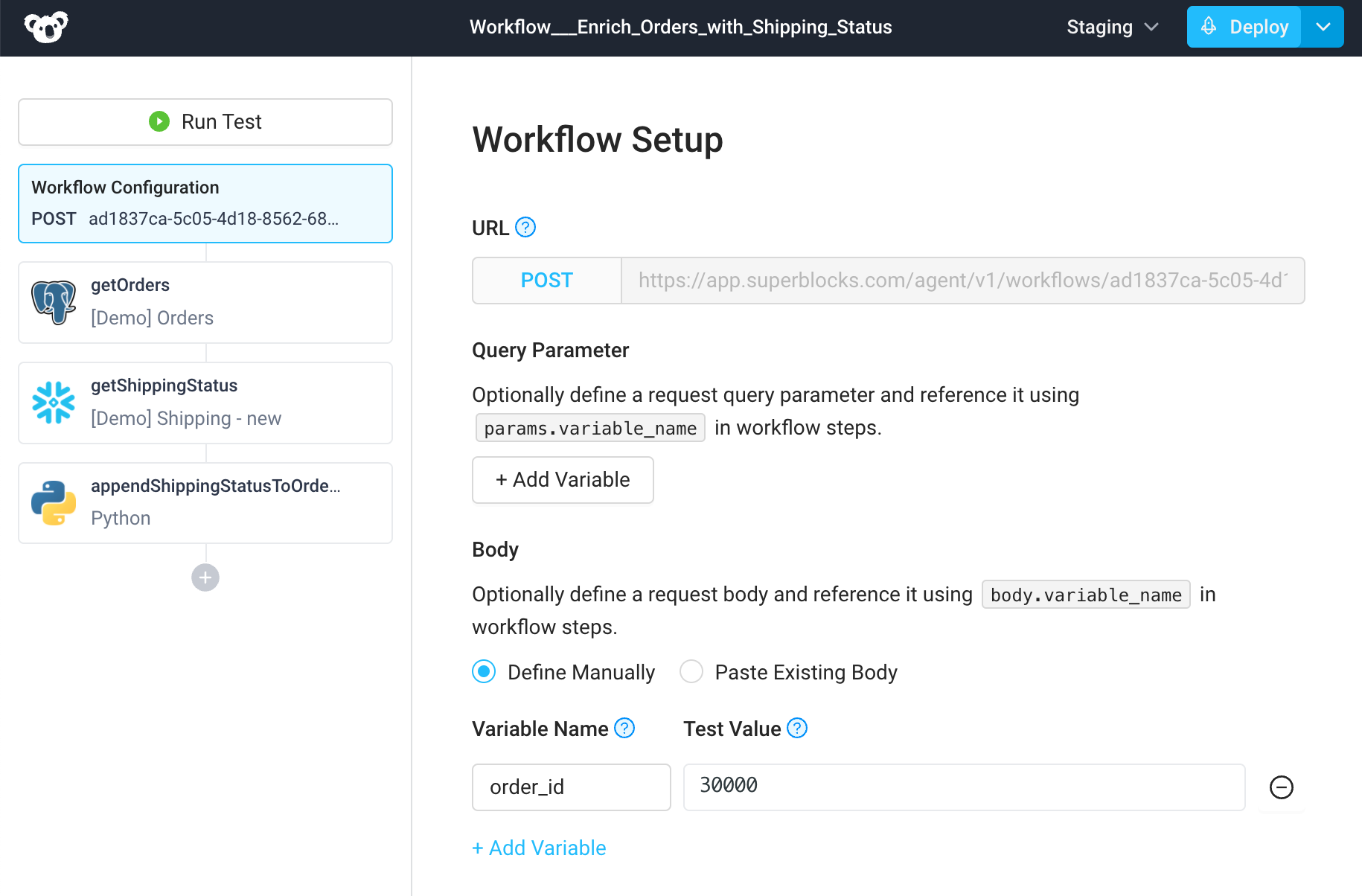
Now when we're building Superblocks Applications, Scheduled Jobs, or even other Workflows, we can reference this workflow as one of the API steps. For example, we have an app that contains an Input component for a user to specify the order ID, and a Table to display the order details and shipping status.
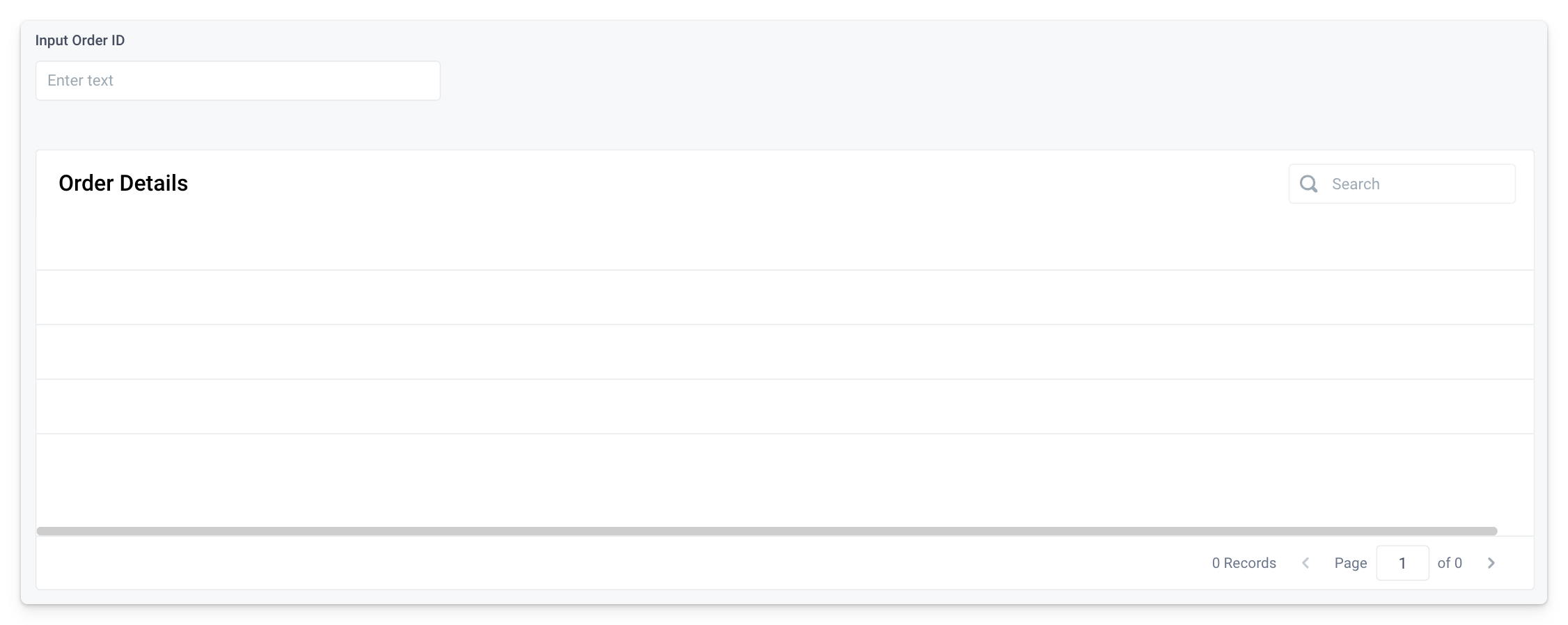
To dynamically populate these components with data, we can create an API that simply calls the workflow we already built. We can pass data from the app components to the workflow request body, as seen with the order_id
taken from an Input component here.
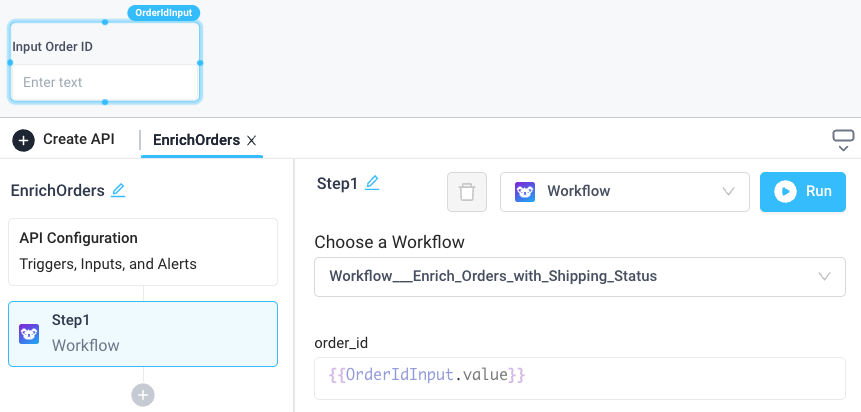
In the User Interface, we can trigger the EnrichOrders
API upon submitting an Input, and bind the Table data to the API response.
When building other Superblocks Applications, Scheduled Jobs, and Workflows that require the same data, we can save time by reusing this workflow and transforming the data as needed for each use case.
Workflow response
When calling a workflow, the response returned to the client contains information about the execution, output, and errors. For example, below is the result of a successful workflow that completed with no errors.
{
"execution": "018df715-5fd0-7cdd-8a51-ef2faf95a33f",
"output": {
"result": "hello world",
"stdout": [
"example printed log",
"another log"
]
},
"status": "STATUS_COMPLETED"
}
In comparison, a workflow that hits any errors returns these in an errors
array.
{
"execution": "018dfadd-d817-767e-abe8-cb1a00d8b8c0",
"errors": [
{
"name": "IntegrationError",
"message": "Error on line 1: name 'time' is not defined ",
"blockPath": "Step2"
}
],
"status": "STATUS_COMPLETED"
}
For an async workflow, the response is returned immediately, and only contains the execution id along with the status.
{
"execution": "018dfade-6724-77de-a7d9-60365ad9486a",
"status": "STATUS_EXECUTING"
}