Custom Components FAQ
Where do I define my component's properties and events?
Properties and events are defined the config.ts file. This file is the source of truth for your component configuration.
How can I define a default value for my component's properties?
When working with properties, you might want to define a default value that is set in the properties panel and is passed to each new instance of a component when it is dragged onto the canvas. Default values for properties can be defined by setting a defaultValue in config.js
:
propertiesPanelDisplay: {
// other settings ...
"defaultValue": "My default value"
}
Why would I expose a property in the properties panel?
Exposing a property in the properties panel allows you to let developers building apps in Superblocks to set the value for the component. Some common examples include:
- Configuration Options - for example, if a component took a
Background Color
property which dictated the background color of the component - Data - for example, a
Table Data
property which includes the data to be displayed in the table
Learn more about configuring your properties panel display.
Why wouldn't I expose a property in the properties panel?
It is common to hide a property from the properties panel when you do not intend for developers building apps to set the property up-front. Typically, the values for these properties are calculated/derived based on other properties and/or user interaction. For example:
- A
Search Radius
property which is calculated based on a user's input on a map - A
Current Expanded Row
property for a table which is set when a user clicks to expand a row
Should I make my property externally readable?
Making a property readable externally ensures that other components and APIs can read the value of the property, which is commonly the desired behavior. In some cases, you may only be using a property for the component's configuration, or you may be using it as an interim to derive some other property; in these cases, it may be cleaner not to make the property externally readable.
Should I make my property externally settable?
Making a property readable settable allows other components and APIs to set (or reset) the value of the property. In some cases, this may not be desirable; for example, a display configuration property which should only be set on initialization and should not be manipulated by usage of the application.
How do I update the values of my component's properties?
In order to update the values of your component's properties, use the provided updateProperties
method.
What is a reserved property?
While developing your component, you may have attempted to create a property and received an error stating that the property path must not match a reserved property path.
In Superblocks, there are a small subset of properties which are maintained internally and shared across many components, including Custom Components. In order to avoid conflicts, these properties are considered "reserved", meaning a Custom Component cannot have its own property which uses the same path. The full list of reserved properties are:
default_* (any path beginning with "default_")
ENTITY_TYPE
bindingPaths
bottomRow
dynamicBindingPathList
dynamicTriggerPathList
gridColumns
gridRows
height
isLoading
isVisible
leftColumn
parentId
rightColumn
snapColumns
snapRows
top
topRow
type
widgetId
widgetLastChange
widgetName
width
How do I import libraries from the web?
In order to import libraries from the web, you can simply use your favorite package manager (i.e. NPM, Yarn, PNPM) and run the install command in your application directory.
All dependencies are managed out of the package.json
file like any other React project.
For an example import, check out how we imported an icon component from Ant Design in the Quickstart guide
How do I import from my company's internal component library?
The best approach to import from your company's internal component library is to use a private registry. If your organization does not already have a private registry, you can learn how to create one on NPM here.
Then, you can simply add a dependency to your package.json
file as you would in any other React project.
Alternatively, you can leverage the symbolic link approach documented below.
How do I share components across Superblocks applications?
Currently, Custom Components are scoped to a single app in Superblocks. Superblocks is working on a native feature to share components across your organization.
In the meantime, if you would like to share components across apps, you can manage your component code out of a single directory using symbolic links. Mac OS "aliases" do not work!
This approach allows you to manage your custom component out of a centralized directory. You'll then link the component in each app's local directory to that central diretory.
Each time you wish to make the component available to an application, you'll need to run the following command within the local directory of that app.
ln -s [PATH_TO_COMPONENT]/[COMPONENT] components/[COMPONENT]
For example, for a component named MyComponent
whose code and config files are located in a central directory [PATH_TO_COMPONENT]/MyComponent/
, run this symlink command from your local app directory:
ln -s [PATH_TO_COMPONENT]/MyComponent/ components/MyComponent/
Note, the component filename must match exactly in the linked directory
Be sure to install any necessary shared dependencies in your package.json
, and then run superblocks components watch
to test if your component works correctly.
Now, your local directory for that component will reference the directory you've linked it to. You'll then need to upload that component as you would any other component, in order to make it available to your app in Superblocks.
If you update the component files that are referenced via symlink, you will have to reupload any dependent components by running superblocks components upload
.
How do I turn on local development mode for Custom Components?
In order to enable local development mode, you need to run your local development server with the superblocks components watch
command and enable Local Dev Mode in your application.
Can I include parameters when triggering events from my React code?
No - Event Triggers expect no arguments, and thus are not parameterized.
Instead, you should simply track the value you wish to propagate as a property of the component, and update this property accordingly. Then, when building out the event handler in Superblocks, you can access the value of that property using the appropriate binding.
For example, if I was building a task list, and I wished to access the task being deleted in my event handler, I could:
- Create a property
latestDeletedTask
- Update this property using
updateProperties
in my React code when a task is deleted - Access the property in my event handler with a binding like
{{ToDoList1.latestDeletedTask}}
How do I detect when some data my component is dependent upon is loading, and what should I do?
In Superblocks, it's common for your component to depend on the output of an API. Often that API will take some time to load, which will result in your component temporarily receiving null
as the value for that property.
In order to handle this case, Superblocks provides the useSuperblocksIsLoading
hook, which indicates whether some data the component depends on is loading.
Commonly, you'll want to handle this case by displaying some loading state to the user.
Note: You should also be sure to handle cases in which a property is null
gracefully, since it's possible that the property may be null while isLoading
is false
- for example, if a developer does not provide a value for a property in the properties panel.
How do I access the component's dimensions in the app, so I can support dynamic behavior like scrolling and responsive layouts?
You may want your component to behave differently based on its size on the screen - for example, to support scrolling and responsive layouts.
In order to handle this case, Superblocks provides the useSuperblocksWidgetSize
hook, which provides an object containing the component's size on the canvas.
Can I set the size of my component when it is initially dragged onto the app canvas?
Yes, you can use the gridDimensions
property in the config.ts file to set the initial grid dimensions.
Why are arrow keys clicked inside my component moving the component's position?
Events from your component are bubbled up to the Superblocks App editor by default. You can use event.stopPropagation()
on keydown events to prevent this behavior. For example:
<input
onKeyDown={(event) => {
event.stopPropagation();
}}
/>
Why doesn't the drag & drop interaction in my component work as expected?
This issue is also related to the fact that events from your component are bubbled up to the Superblocks App editor by default. You can use event.stopPropagation()
on mousedown events to prevent this behavior. For example:
<MyComponent
onMouseDown={(event) => {
event.stopPropagation();
}}
/>
Can I build popovers, modals, slideouts, and other components which expand outside the component boundary?
Yes! The App Builder is architected so that the entire app canvas is inside of an iframe. This architecture ensures that Superblocks can securely provide you access to style the entire iframe, unlike many other offerings which limit styles to a dedicated iframe for each component. Thus, you can control the entire iframe, enabling you to build components which expand outside the component boundary. Check out the example full-screen text editor modal Custom Component below.
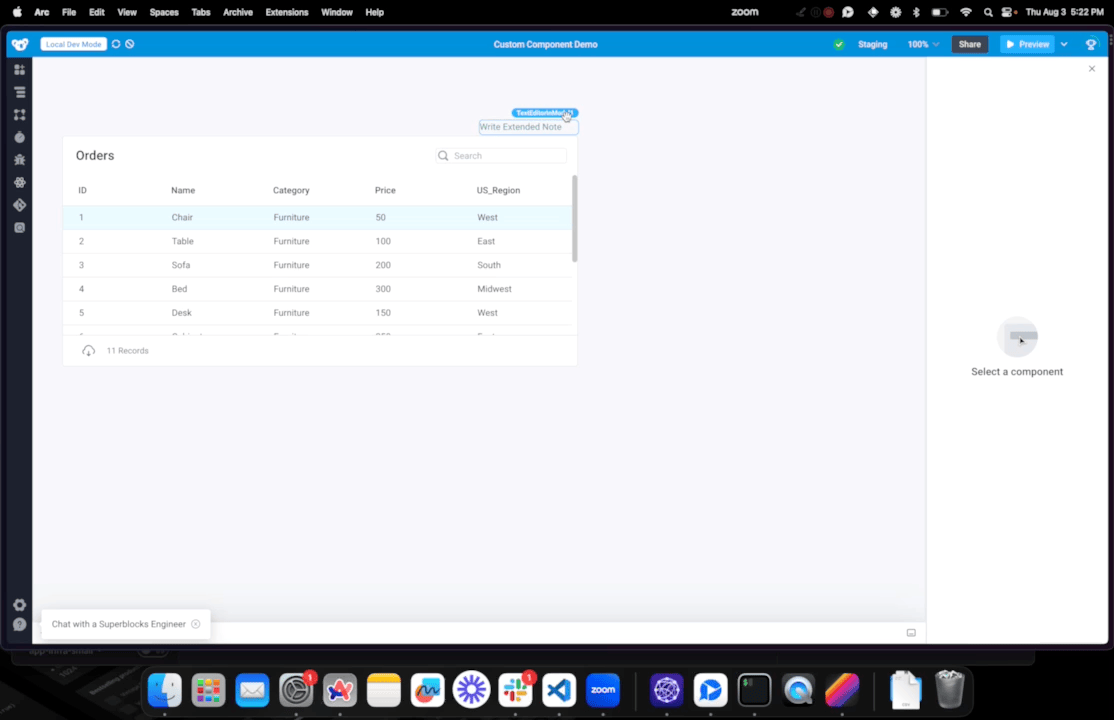
What versions of Node are supported?
The Superblocks CLI supports Node LTS versions, which are documented by Node here. If you run into errors using the CLI, please check your Node version (by running node -v
) to ensure that your version is compatible with the Superblocks CLI.
What versions of React are supported? How do I use React 17?
Custom Components defaults to React 18, but React 17 is also fully supported. In order to switch to React 17:
- Update the relevant dependencies in your
package.json
(dependencies.react
,dependencies.react-dom
,devDependencies.@types/react
,devDepenencies.@types/react-dom
) - Run
npm install
What happens if Superblocks changes the Custom Components system? Will my components and/or apps break?
While uncommon, we sometimes need to introduce incompatible changes; for example with an update to the Custom Components React API or config.ts
file format.
In these cases, Superblocks guarantees that previously uploaded components will remain safe. These components will continue to function as expected across all modes (edit, commits, deployed) of your application.
The next time you develop your component, you will be required to upgrade the component code. This upgrade will be prompted by the CLI and may be partially automated and partially manual. We will provide accompanying documentation for any manual changes required.