Timers
Timers have moved to the Navigation panel since the recording of the video below.
Timers allow you to invoke behavior repeatedly at a timed interval. When the timer interval expires, the timer fires an event, which you can respond to using the onFire
event handler.
Auto-refresh a dashboard with a timer
We can refresh any component's data automatically with a timer. For this example, we'll use a chart.
Start by dragging the Chart component from the Components panel to the empty canvas. Set the Chart Header to Number of Signups
, the X axis title to Day of month
and the Y axis title to User Signups
.
To populate the chart from an external source, we can use the Superblocks API builder. In this case, we will use a JavaScript step that generates sample user signup data for us.
- Create a new backend API with a JavaScript step
- Rename API1 to
getSignups
- Copy and paste the following code to the JavaScript step
data = [];
for(var i=1; i<10; i++){
const date = i;
const value = Math.floor(Math.random() * 60) + 1;
point = {};
point.date = date;
point.value = value;
data = [point, ...data];
}
return data;
In the application user interface, click on the chart to open the properties panel. To connect the results from getSignups
API to the chart, replace the example JSON with {{getSignups.response}}
in the Chart Data field and Run the API. Finally set Chart Type to Line Chart
.
Turn off the Loading Animation setting for a smooth animation.
Next, link the Chart Component to a Timer that updates it at a set interval.
- Go the Navigation panel and click the + sign next to Timers
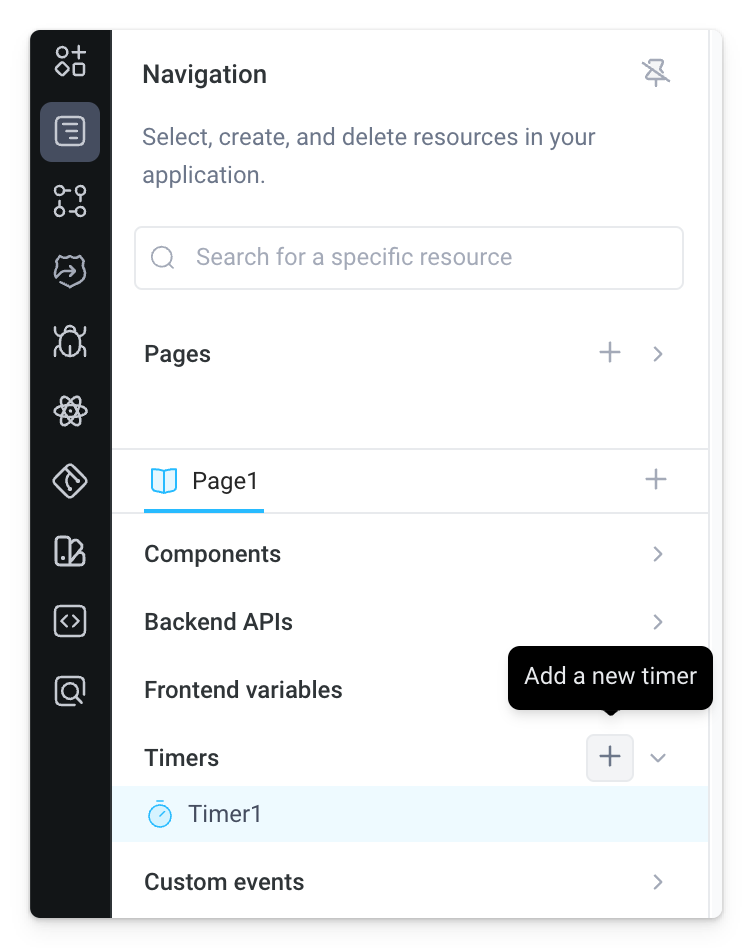
- Select the timer to configure the Interval (5 seconds) and onFire event hander (run the
getSignups
API)
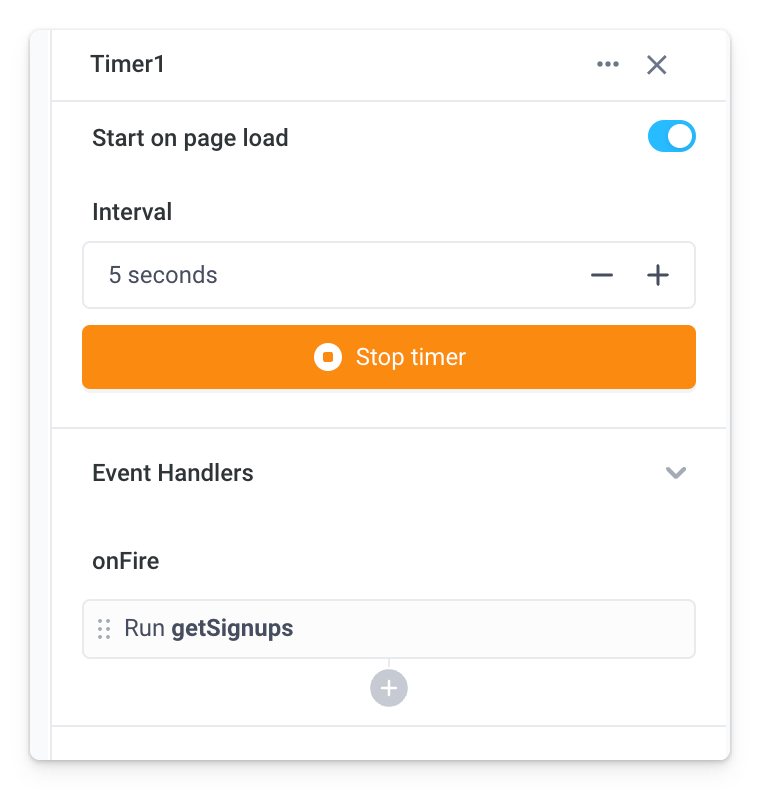
That's it, your User Signup Dashboard will now automatically update with the latest signups every 5 seconds.
Starting and stopping timers
Starting timers on page load
You can control whether a timer starts on page load by toggling the switch in the timer's properties panel.
Starting and stopping from component triggers
You can hook up any component trigger (like a Button component's onClick
) to start and stop timers. Just choose the Start/stop Timer option from the triggers menu.
You can also start or stop timers from code inside of a Run JS action. See Programmatic usage (using code to control timers)
Starting and stopping timers from the UI
You can start or stop a timer from the UI by going to the State Inspector panel and clicking the start/stop button next to the timer, or by selecting the timer and using the button in the properties panel.
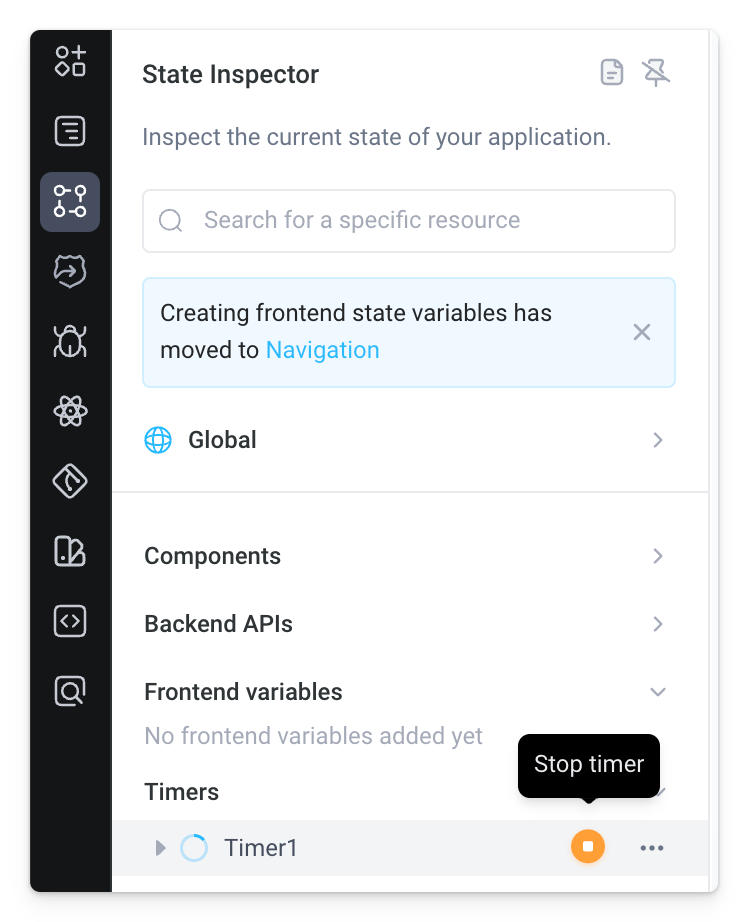
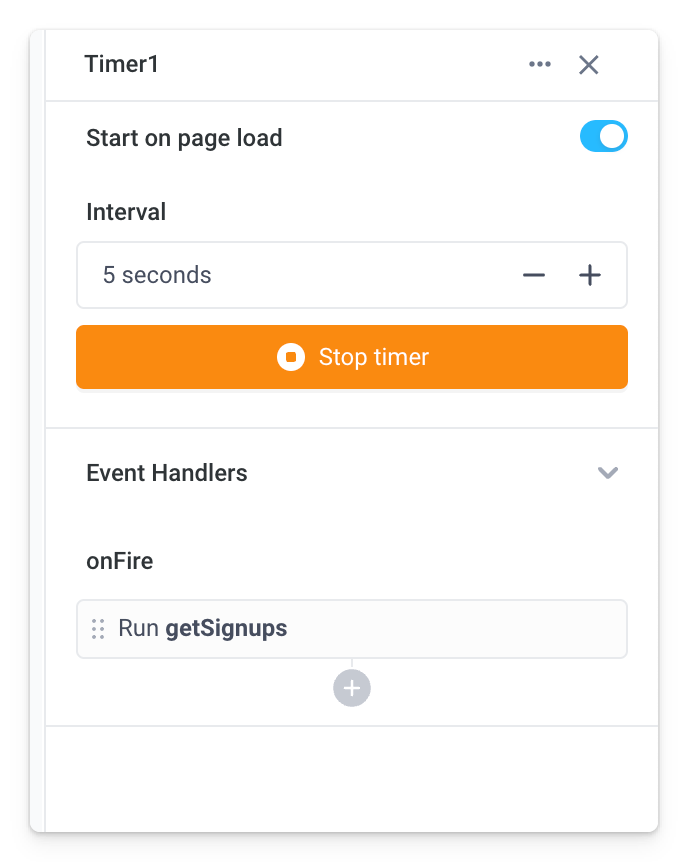
Programmatic usage (using code to control timers)
You can start or stop a timer using code in Run JS (in browser) actions by referencing its name and calling start
or stop
.
Timer1.start();
Timer1.stop();
You can also "toggle" a timer without needing to know if it's started or stopped by calling toggle
.
Timer1.toggle();
Properties
Timer properties can be accessed from other frontend components and backend APIs by adding the name of the timer, and dot referencing the property. For a timer named Timer1:
Property | Description |
---|---|
Timer1.isActive | Access whether the timer is currently running |
Troubleshooting
How do I not show the loading animation when my API is running?
Turn off the Loading Animation setting in the properties panel of your component to update your the component without showing a loading animation.