Navigation
To connect the pages in your application, you will need to establish Navigation between them. There are several methods to facilitate this navigation.
Multi-page Superblocks apps employ a single-page app architecture to ensure quick and smooth page transitions, typical of modern web applications.
Link component
The best way to facilitate navigation between pages is using the Link component. This component enables easy linking to other pages in your app.
Because the Link component renders a standard HTML hyperlink (anchor element), it provides all the standard browser behavior around links such as
- Cmd/Ctrl + Click to open in new tab
- Right-click context menu to open in new tab, copy link URL, etc
- Link previews in browser
To link to another page in your app, simply:
- Drag on a Link component
- Ensure the Link to property is set to Page
- Select the relevant page to link to
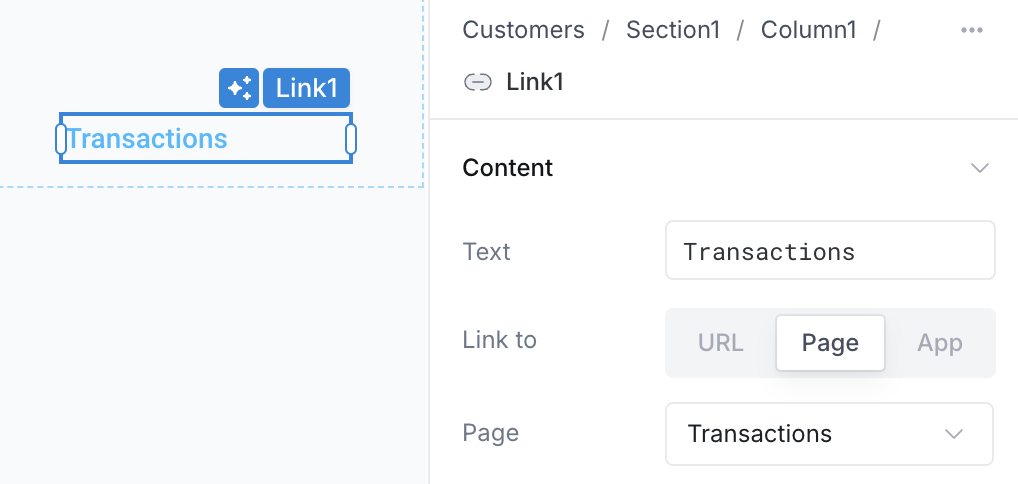
Navigate to page action
You can also navigate to pages in your app using the Navigate to page action.
The Navigate to Page action can be connected to any event handler to initiate navigation to another page. This action is useful if you want to navigate to a page from a component that does not natively support links, such as a button.
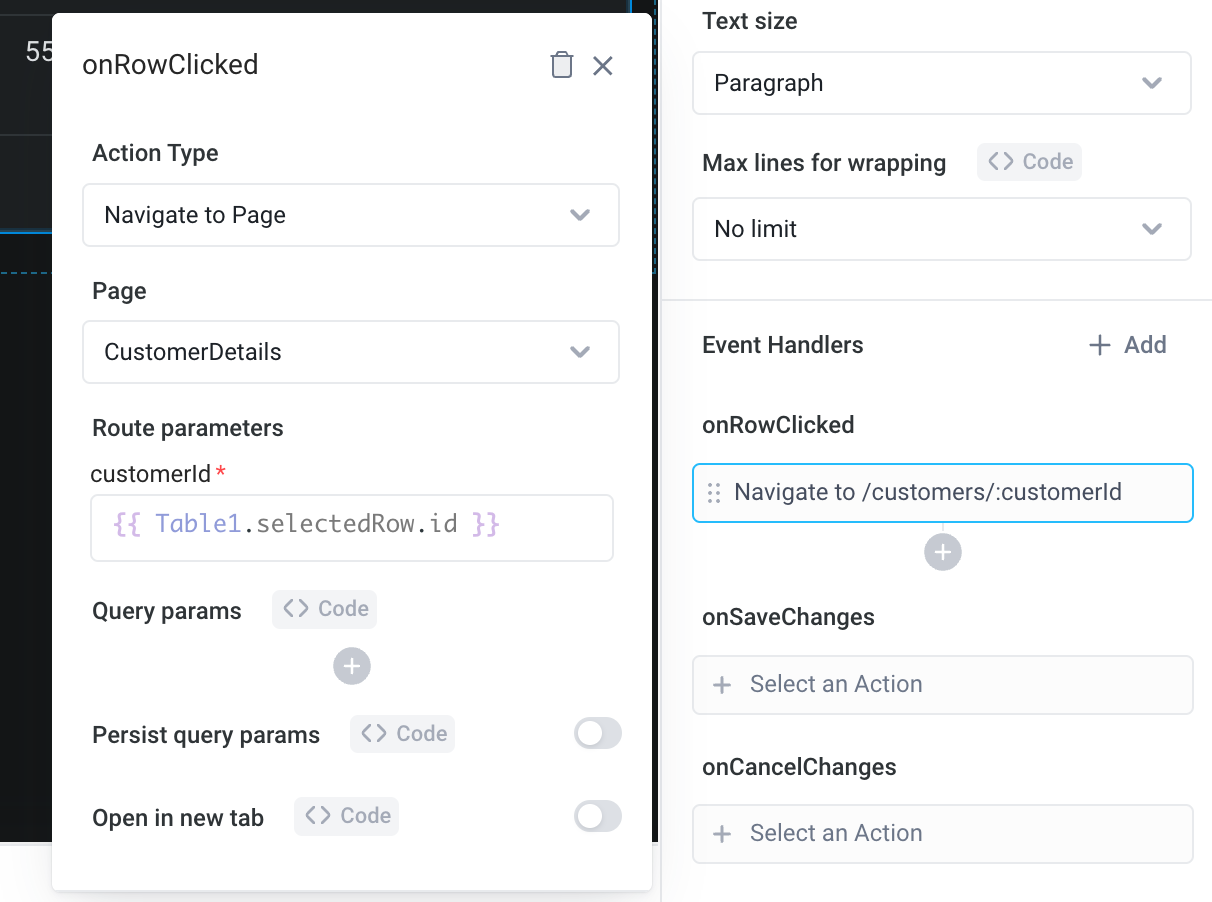
Example - Navigating to a CustomerDetail page
In a multi-page app, a common interaction involves having one page for listing resources (List Page) and another for viewing specific details (Detail Page). For instance, consider a Customer List page displaying users, with the URL route /customers
.
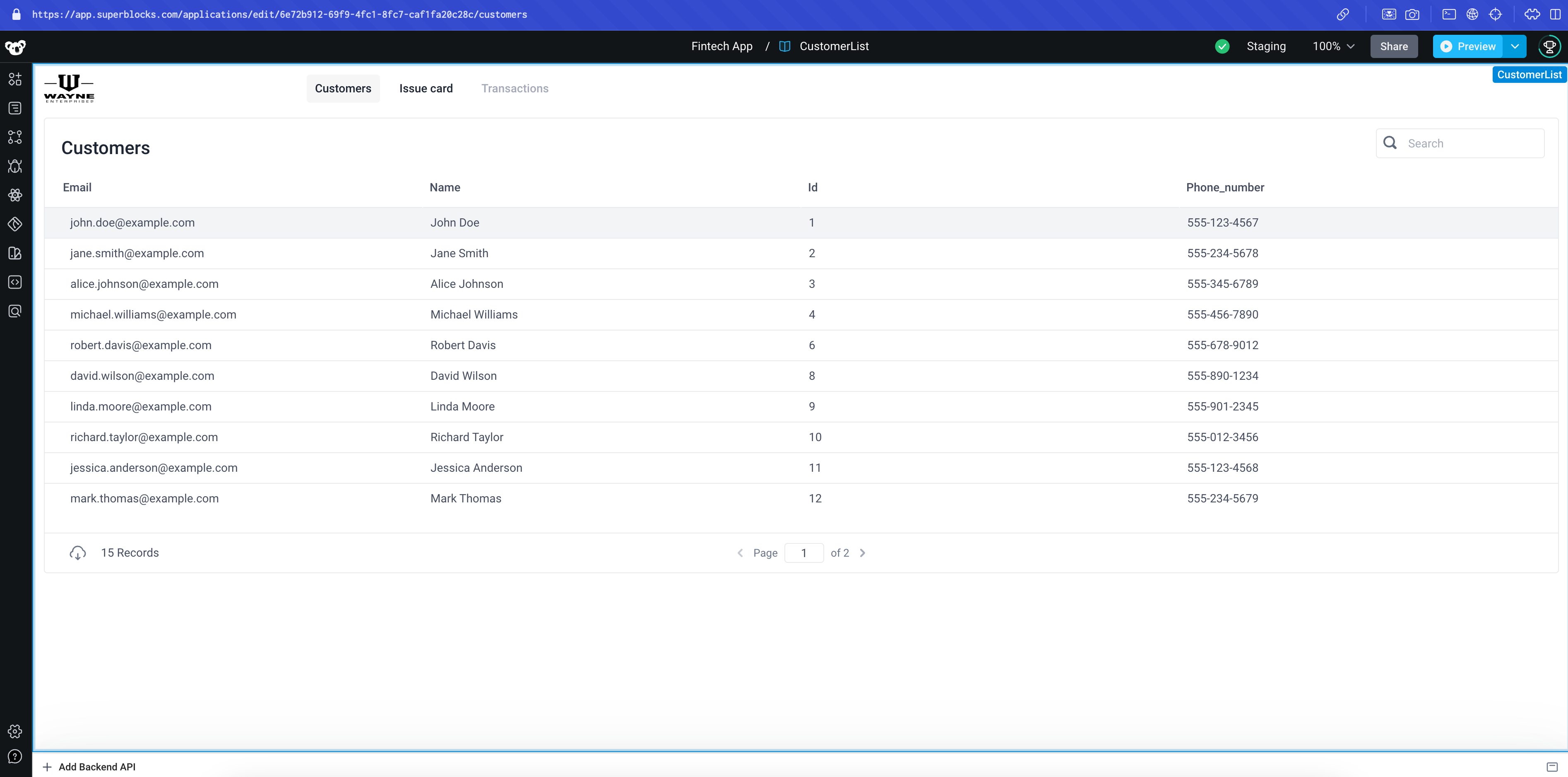
Additionally, there's a Customer Detail page with a dynamic route /customers/:customerId
. To enable navigation from the list to the details page, clicking on a customer row in the table should lead to the Customer Detail page for the selected customer.
To acheive this, access the table's onRowClicked
event handler and configure a Navigate to page action. From there, pass {{TableName.selectedRow.id}}
as the customerId route parameter.
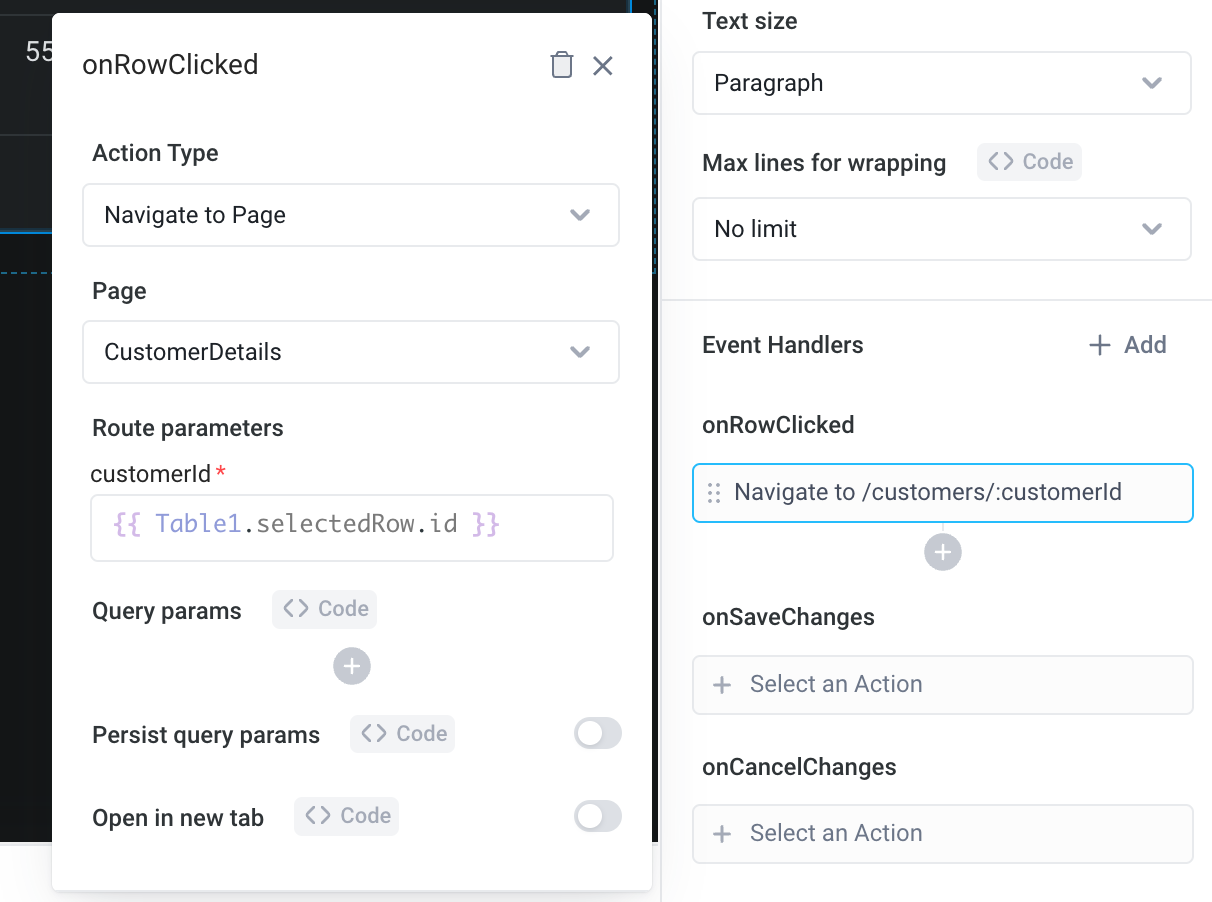
Now, clicking on a specific row navigates to /customers/:customerId
, where :customerId
represents the ID of the selected item passed as the route parameter to the Customer Detail page.
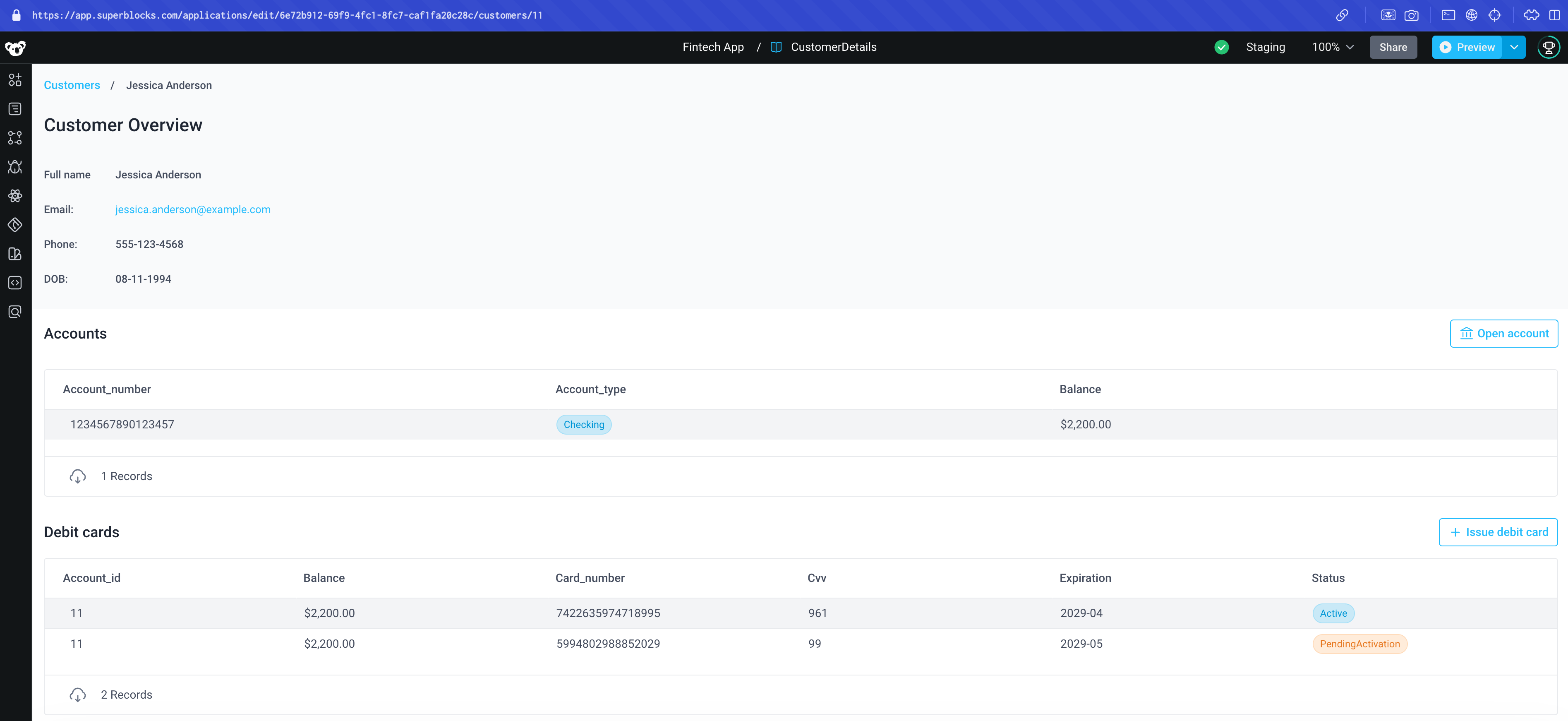
Dynamic navigation
You can easily navigate dynamically to different pages in an application using either the Navigate to URL action or the navigateTo
function in a Run JS action.
When navigating to a different page in your app, it is only necessary to specify the relative path to the page (i.e. the fully resolved route). For example, if the root page is at https://app.superblocks.com/applications/d3c0a8d8-858b-4f4f-a9e1-1b5d3bcbfbd2
, you only need to specify /users
as the URL in order to navigate to the Users page.
To demonstrate dynamic navigation, imagine using a dropdown to navigate between pages instead of the navigation bar shown in previous screenshots.
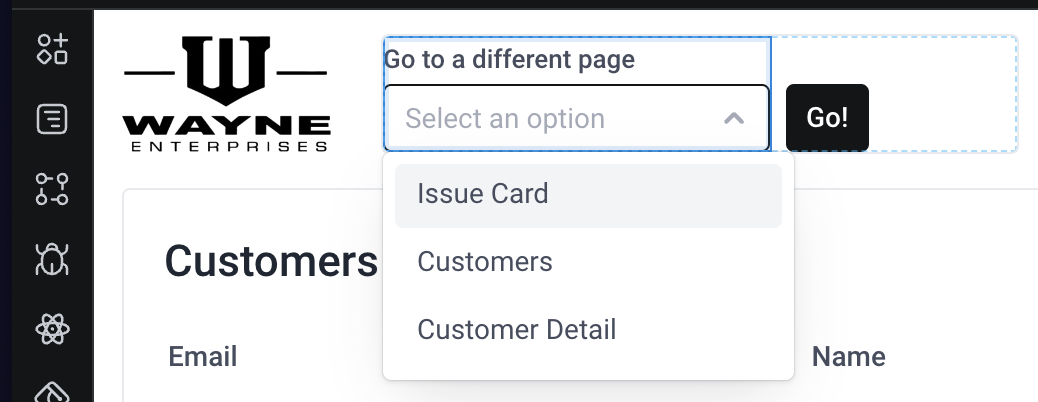
Navigate to URL action
One way dynamic navigation can be achieved is with the Navigate to URL action.
In this case, the Dropdown is configured with the following options data.
[
{
"label": "Issue Card",
"value": "/issue-card"
},
{
"label": "Customers",
"value": "/customers"
},
{
"label": "Customer Detail",
"value": "/customers/{{Table1.selectedRow.id}}"
}
]
Now, when a user clicks the Go! button, the app should navigate to the page selected in the dropdown.
To achieve this, use the button's onClick
event handler and set up a Navigate to URL action. Instead of providing a static URL, simply specify {{Dropdown1.selectedOptionValue}}
as the URL. This approach works well since the options object already contains the relative paths for each of our pages.
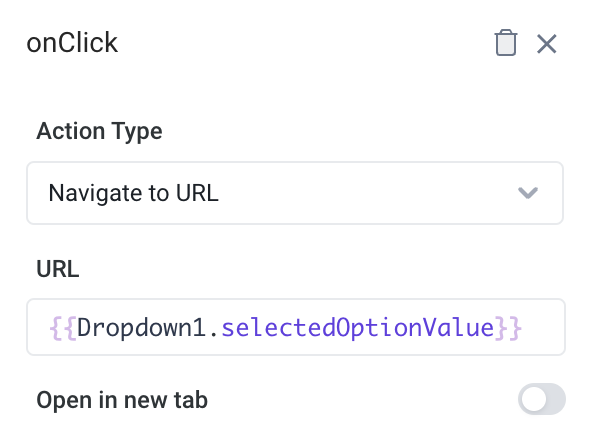
Now, when a user selects the Issue Card option from the dropdown and clicks the Go! button, the Navigate to URL
action will trigger with the URL set to /issue-card
, navigating the user to corresponding page.
Navigation in Run JS
Alternatively, the navigateTo
function can be used in the Run JS action to dynamically navigate to different pages in the app. This approach is useful for implementing more complex logic, such as a series of conditionals to construct URLs differently.
For this example, consider that the Dropdown options data is structured as:
["Issue Card", "Customers", "Customer Detail"]
In this case, additional logic is needed to determine the destination for the navigation. While this could be handled within a binding in the Navigate to page
action, running it within the RunJS
action offers a more ergonomic approach.
let selectedPage = Dropdown1.selectedOptionValue;
switch (selectedPage) {
case "Issue Card":
navigateTo(`/issue-card`);
break;
case "Customers":
navigateTo(`/customers`);
break;
case "Customer Detail":
navigateTo(`/customers/${Table1.selectedRow.id}`);
break;
default:
showAlert("Page not found", "warning", 4, "top");
}
Navigation and events
Whenever you navigate within a Superblocks app, the pageLoad
event will be triggered on the destination page. Consequently, any APIs set to run onPageLoad
will execute after navigation.
This behavior persists even when within the same page. For example, updating the route or query parameters using the Navigate to page action will trigger the pageLoad
event, causing any associated APIs to re-run.
Building a standard navigation bar
Most web apps have a standard navigation used to switch between pages. For example, think of the sidebar navigation in Shopify or the top navigation bar in Github.
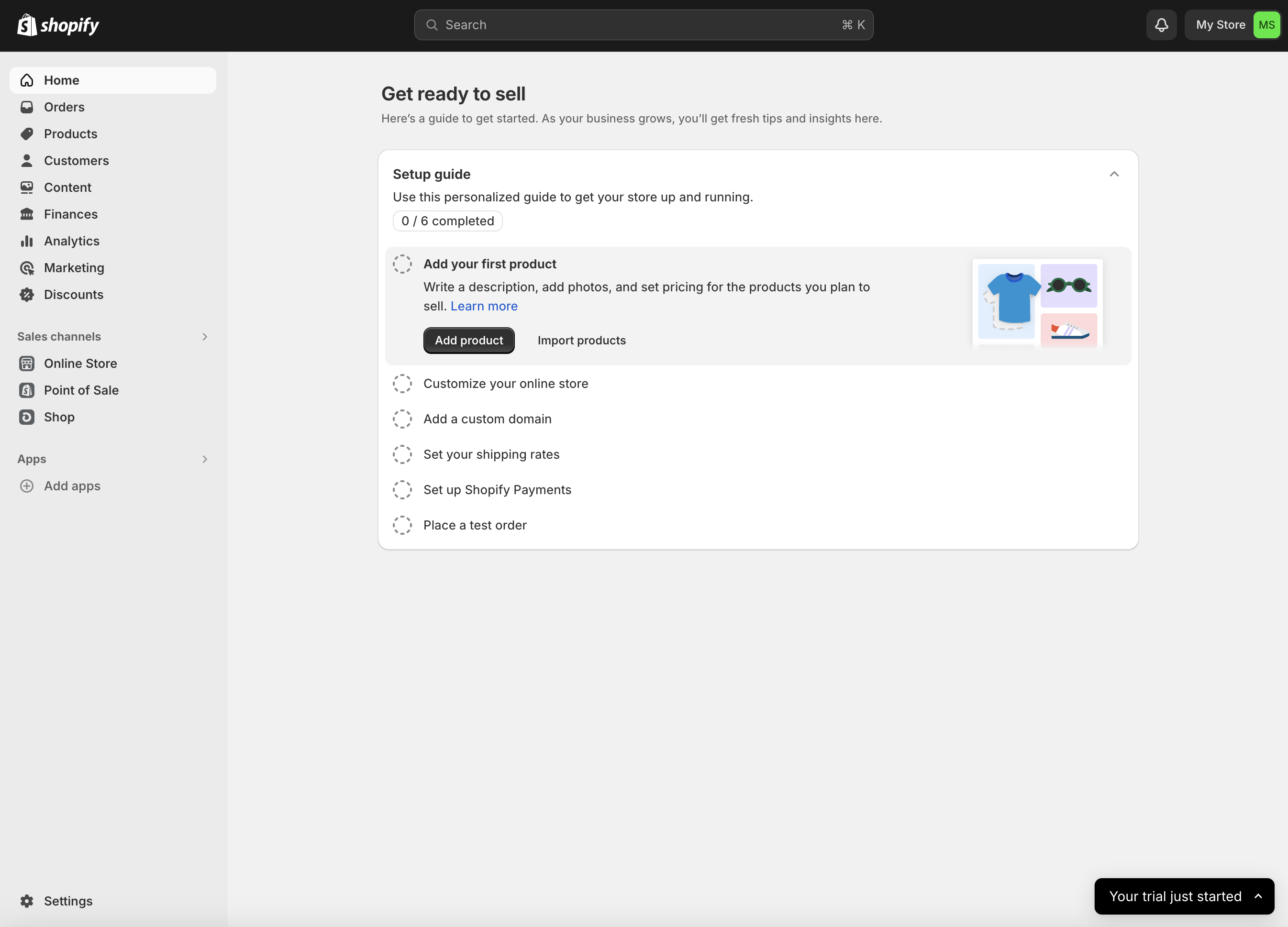
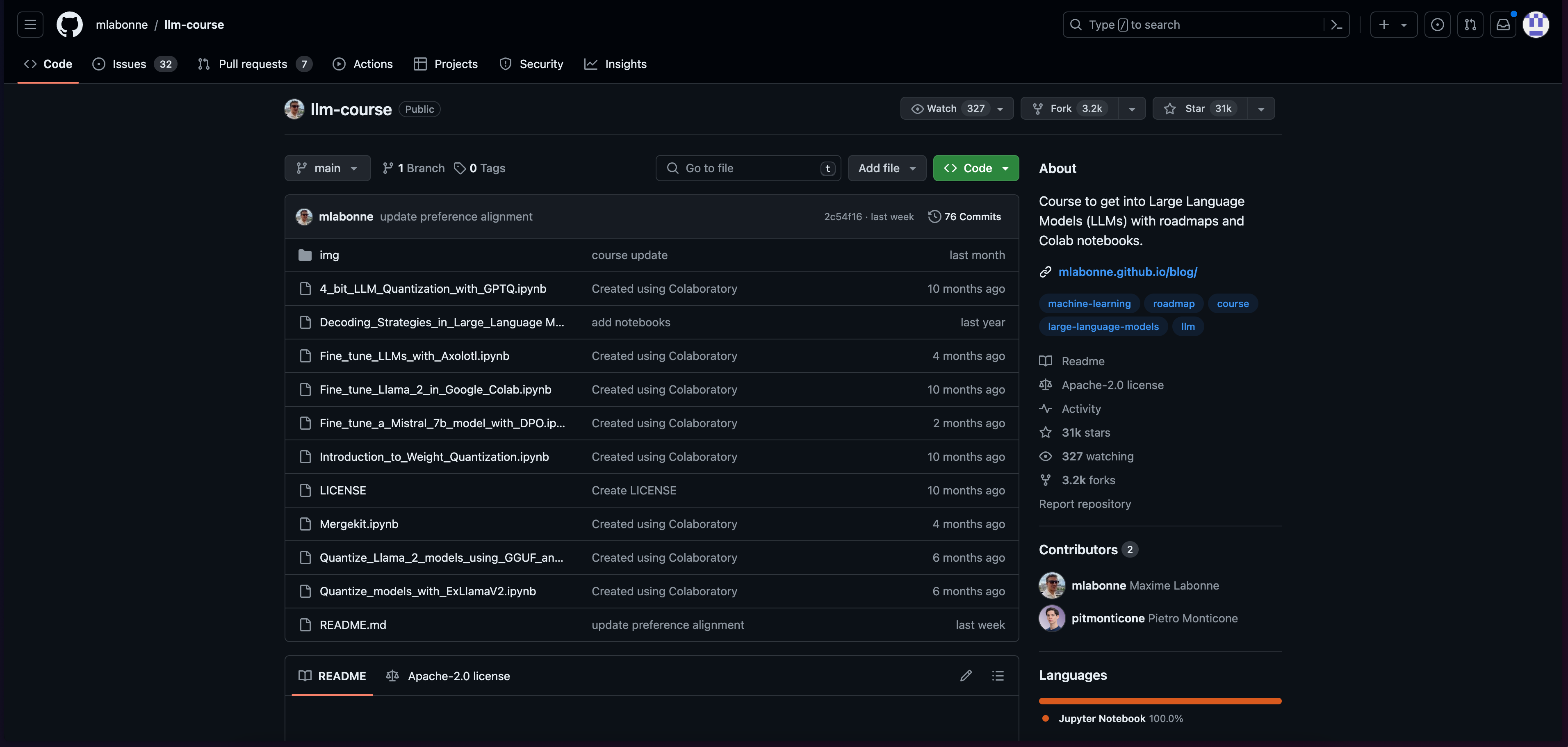
In Superblocks, you can easily build your pixel-perfect Navigation using a combination of Layouts and Multi-Page.
Using UI Templates as the starting point
To get started, you can simply grab a sidebar navigation or top navigation bar from the UI Templates library. These navigation bars come out-of-the-box with Link and Menu components, as well as the proper layouts.
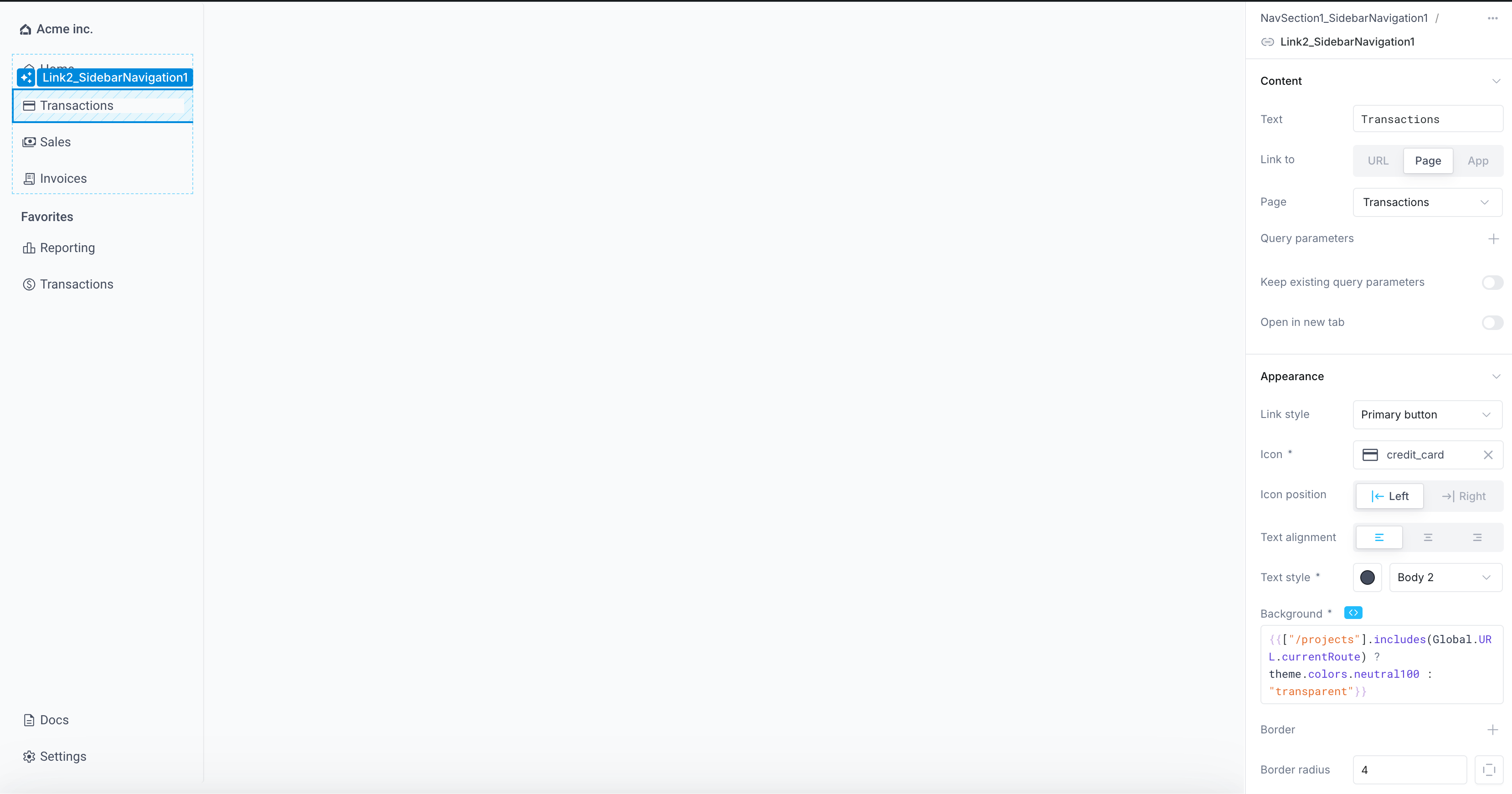
You can simply add, remove, and customize the existing links in the template to link to the relevant pages in your app.
Duplicating navigation to other pages
Once you've built your navigation, you can easily copy it over to other pages. Simply select the Section, Column, or Container, copy it clipboard, and paste it over to the new page.