Limit access to UI components and Backend APIs
Customize the UI of your application based on the current users permissions using the Global.user
object.
Authorization example
Lets say we're building a payment processing application that our support & finance team can use to manage customer orders, issue invoices, and report on payments. Only our level three (L3) support agents are authorized to issue order refunds.
We want to enforce this authorization in two ways:
- Disable the
refundButton
in the UI - Add an authorization check on the
refundOrder
backend API so only L3 agents can run the API
Disable/hide UI elements
Using the Disabled
and Visible
properties of components, you can customize the UI of your application based on the permissions you've given to end-users.
In our example above, we want to disable the refundButton
so it's only accessible to our L3 agent team. To do this, we'll add the following code to the Disabled
property of the refundButton
, making the button conditionally disabled based on if the current user is a member of the "L3 Agents" user group.
{{
!Global.user.groups
.map(item => group.name)
.includes("L3 Agents")
}}
Alternatively, we could hide the button completely using the Visible
property of the refund button. Now when the end-user isn't a member of the L3 Agent group, they won't see the refund button at all.
Limit access to APIs
You can limit which of your app's APIs users are allowed to execute by adding authorization checks to your APIs. When enabled, the Superblocks agent will perform authorization checks for you before executing your APIs. If the logged in user isn't allowed to execute the API, the request will be rejected with an authorization error.
To configure API authorization:
- Open the Backend API you want to limit access to
- Click into the API's trigger
- Change the Permissions of the API to Authorized users
- Add the users or user groups allowed to execute the API
Add custom authorization checks in backend code
You can alternatively implement authorization checks yourself in your backend API's code.
In this case, Superblocks won't perform authorization checks for you, but you will have more control and flexibility over how authorization is performed and the error messages returned to users.
Lets say for example, we want to update our refund functionality so that:
- Members of the L3 Agents group can issue refunds of any amount
- Members of the L2 Agents group can issue refunds up to a certain limit
- If the refund amount exceeds an L2 agent's limit, they can escalate the request to an L3
We can implement this logic using Conditional and Throw control blocks in our API.
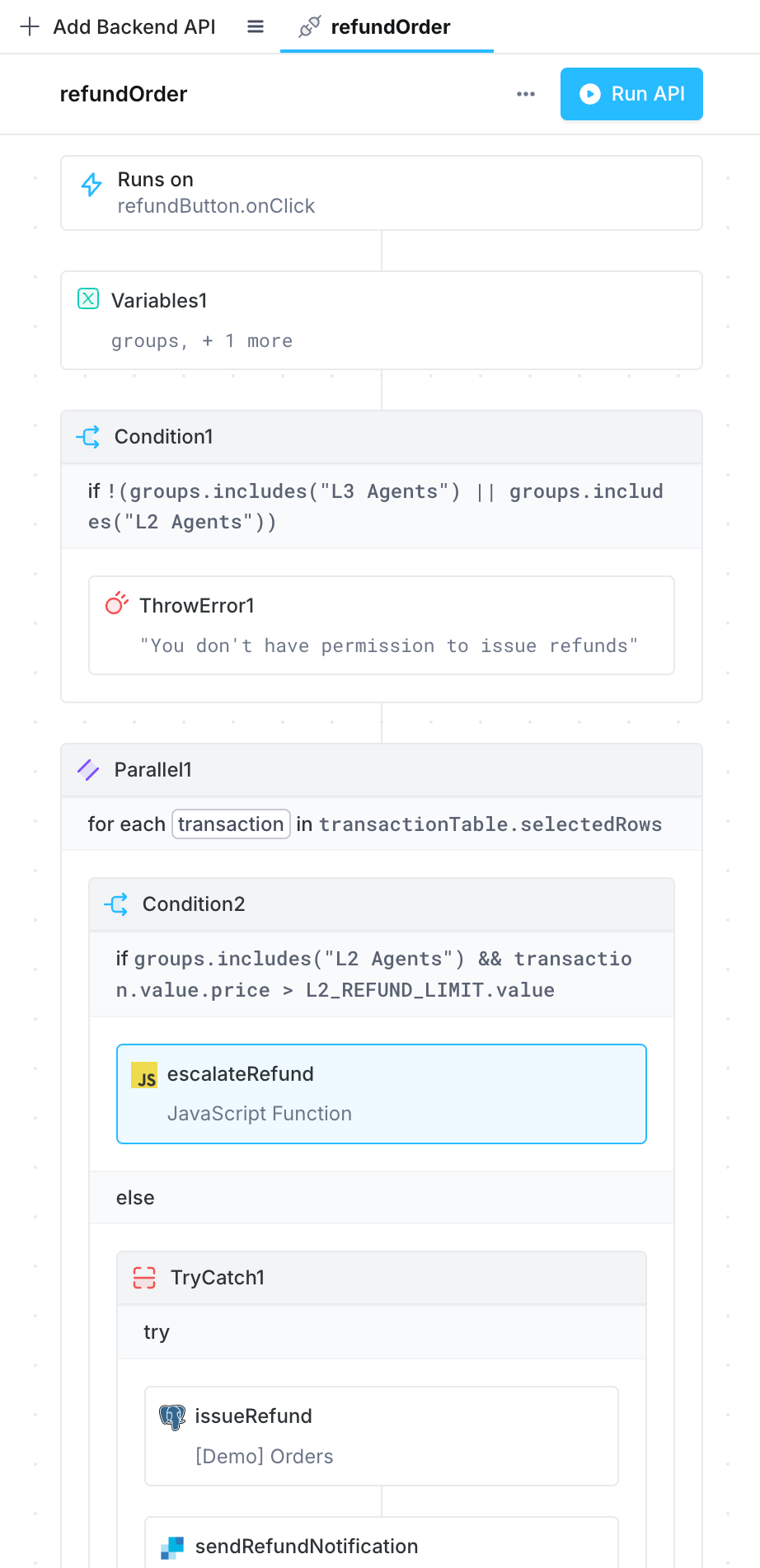
In this example, the conditionals evaluate which group users are in to decide if an authorization error is thrown (ThrowError1
), or if the refund request is rejected and added to a list of refunds that the agent can request be escalated (escalateRefund
).